python判断字符串是否包含abc
时间: 2023-08-11 08:08:31 浏览: 125
可以使用Python中的`in`关键字来判断一个字符串是否包含另一个字符串。示例如下:
```
s = "abcdefg"
if "abc" in s:
print("s包含abc")
else:
print("s不包含abc")
```
输出结果为:"s包含abc"。如果s中不包含"abc",则输出结果为:"s不包含abc"。
相关问题
python 判断字符串是否包含指定字符串
以下是两种判断字符串是否包含指定字符串的方法:
1. 使用in运算符(推荐使用)
```python
def demo():
str1 = "b"
str2 = "abc"
if str1 in str2:
print("存在")
else:
print("不存在")
demo()
```
输出结果为:存在
2. 使用find方法
```python
def demo():
str1 = "a"
str2 = "abc"
if str2.find(str1) >= 0:
# 包含的话,返回第一次出现的位置,位置下标是从0开始,没有的话为负数
print("包含")
else:
print("不包含")
demo()
```
输出结果为:包含
用python判断字符串的包含关系
### 回答1:
可以使用 python 中的 in 关键字来判断一个字符串是否包含另一个字符串。
例如:
str1 = "hello world"
str2 = "world"
if str2 in str1:
print("str1 contains str2")
else:
print("str1 does not contain str2")
# Output: "str1 contains str2"
也可以使用 `str.find()` 方法来判断,返回值为-1则不包含
例如:
str1 = "hello world"
str2 = "world"
if str1.find(str2) != -1:
print("str1 contains str2")
else:
print("str1 does not contain str2")
# Output: "str1 contains str2"
### 回答2:
在Python中,可以使用以下方法来判断字符串的包含关系:
1. 使用`in`关键字:使用`in`关键字可以判断一个字符串是否包含在另一个字符串中。例如,使用`"abc" in "abcdefg"`,如果字符串"abcdefg"中包含子字符串"abc",则返回`True`;否则返回`False`。
2. 使用`find()`方法:字符串对象的`find()`方法可以用来查找一个子字符串在另一个字符串中的索引位置。如果找到,返回子字符串的起始索引,否则返回-1。例如,使用`"abcdefg".find("abc")`,如果字符串"abcdefg"中包含子字符串"abc",则返回2(起始索引为2);否则返回-1。
3. 使用`startswith()`和`endswith()`方法:字符串对象的`startswith()`方法可以用来判断一个字符串是否以指定的子字符串开头;`endswith()`方法可以判断一个字符串是否以指定的子字符串结尾。这两个方法都返回布尔值。例如,使用`"abcdefg".startswith("abc")`,如果字符串"abcdefg"以子字符串"abc"开头,则返回`True`;否则返回`False`。
总之,以上就是判断字符串包含关系的几种方法。根据具体需求可以选择使用哪种方法来判断字符串的包含关系。
### 回答3:
在Python中,我们可以使用in关键字来判断一个字符串是否包含另一个字符串。这个关键字的语法是:
```
判断字符串1 in 字符串2
```
如果字符串1包含在字符串2中,则返回True;否则返回False。
例如,我们有两个字符串s1和s2:
```
s1 = "hello"
s2 = "hello world"
```
我们可以使用in关键字判断s1是否包含在s2中:
```
if s1 in s2:
print("s2包含s1")
else:
print("s2不包含s1")
```
运行结果将会是:“s2包含s1”,因为字符串"hello"是字符串"hello world"的一部分。
除了in关键字外,Python还提供了startswith()和endswith()方法,用于判断一个字符串是否以另一个字符串开头或结尾。这两个方法的语法分别是:
```
字符串.startswith(子字符串)
字符串.endswith(子字符串)
```
这里的子字符串可以是一个字符,也可以是多个字符组成的字符串。如果一个字符串以指定的子字符串开头或结尾,则返回True;否则返回False。
例如,我们有一个字符串s:
```
s = "hello world"
```
我们可以使用startswith()方法判断s是否以"hello"开头:
```
if s.startswith("hello"):
print("s以hello开头")
else:
print("s不以hello开头")
```
运行结果将会是:“s以hello开头”。
综上所述,使用Python判断字符串的包含关系可以使用in关键字、startswith()方法和endswith()方法。
相关推荐
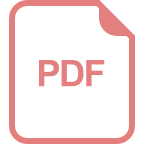
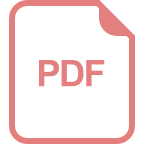












