用Python编写程序设计一个计算器,能进行加法、阶乘、减法、除法、求余、幂等运算,要求每个计算功能通过函数实现,具有结束计算功能
时间: 2024-01-31 12:04:52 浏览: 96
好的,以下是用 Python 编写的计算器程序,实现了加法、减法、乘法、除法、求余、幂以及阶乘等功能,并提供了结束计算的选项:
```python
import math
def add(x, y):
"""加法"""
return x + y
def subtract(x, y):
"""减法"""
return x - y
def multiply(x, y):
"""乘法"""
return x * y
def divide(x, y):
"""除法"""
return x / y
def modulo(x, y):
"""求余"""
return x % y
def power(x, y):
"""幂"""
return x ** y
def factorial(x):
"""阶乘"""
return math.factorial(x)
print("请选择运算:")
print("1、加法")
print("2、减法")
print("3、乘法")
print("4、除法")
print("5、求余")
print("6、幂")
print("7、阶乘")
print("8、结束计算")
while True:
choice = input("请输入您的选择(1/2/3/4/5/6/7/8):")
if choice in ('1', '2', '3', '4', '5', '6'):
x = float(input("请输入第一个数字:"))
y = float(input("请输入第二个数字:"))
if choice == '1':
print(x, "+", y, "=", add(x, y))
elif choice == '2':
print(x, "-", y, "=", subtract(x, y))
elif choice == '3':
print(x, "*", y, "=", multiply(x, y))
elif choice == '4':
if y == 0:
print("除数不能为0!")
else:
print(x, "/", y, "=", divide(x, y))
elif choice == '5':
print(x, "%", y, "=", modulo(x, y))
elif choice == '6':
print(x, "^", y, "=", power(x, y))
elif choice == '7':
x = int(input("请输入一个正整数:"))
print(x, "的阶乘为:", factorial(x))
elif choice == '8':
print("感谢使用本计算器!")
break
else:
print("非法输入,请重新输入!")
```
你可以在终端或命令行下运行该程序,并根据提示进行操作。
阅读全文
相关推荐
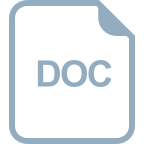
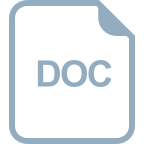
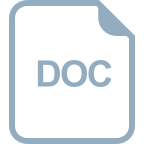


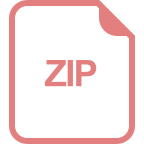
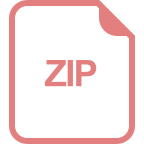
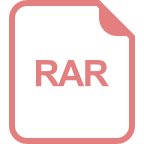
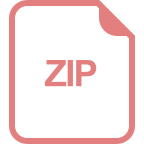
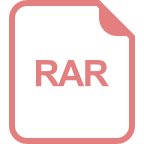
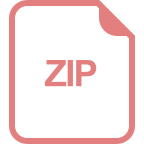
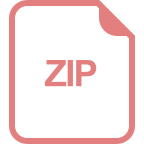
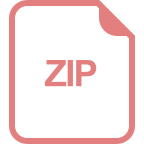
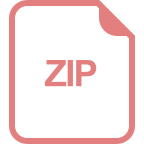
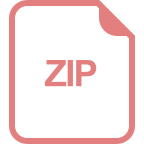
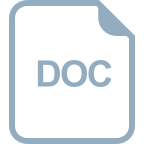
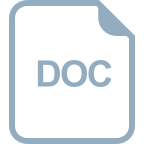
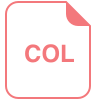
