setting an array element with a sequence
时间: 2023-10-16 11:31:46 浏览: 89
This error occurs when you try to assign a sequence (such as a list or tuple) to a single element of an array. Arrays are a fixed-size collection of elements of the same type, so you cannot assign a sequence to a single element.
For example, the following code would raise this error:
```
import numpy as np
arr = np.zeros(5)
arr[0] = [1, 2, 3]
```
Instead, you need to assign a single value of the same type as the array to the element:
```
import numpy as np
arr = np.zeros(5)
arr[0] = 1
```
If you want to assign multiple values to different elements of the array, you can use slicing:
```
import numpy as np
arr = np.zeros(5)
arr[0:3] = [1, 2, 3]
```
相关问题
python setting an array element with a sequence
The error "setting an array element with a sequence" in Python occurs when you try to assign a sequence, such as a list or tuple, to an individual element of an array or a numpy array. Arrays require individual values for each element, not sequences.
To resolve this error, you can use the `numpy.array` function to convert the sequence into an array. Here's an example:
```python
import numpy as np
my_array = np.array([1, 2, 3]) # Create a numpy array
# Assign individual elements of the array
my_array[0] = 4
my_array[1] = 5
my_array[2] = 6
print(my_array) # Output: [4 5 6]
```
If you want to initialize an array with a sequence directly, you can use the `numpy.asarray` function instead. Here's an example:
```python
import numpy as np
my_array = np.asarray([1, 2, 3]) # Convert the sequence into a numpy array
print(my_array) # Output: [1 2 3]
```
Remember to import the `numpy` module before using these functions.
setting an array element with a sequence.
这个错误通常表示您正在尝试将一个序列(如列表或数组)赋值给单个数组元素,而不是将其作为整个数组赋值。例如,假设您有以下代码:
```
my_array = [0, 0, 0]
my_array[0] = [1, 2, 3]
```
这将导致 "setting an array element with a sequence" 错误,因为您尝试将列表 [1, 2, 3] 分配给 my_array 的单个元素 my_array[0],而不是将其作为整个数组赋值。要解决此错误,您需要确保分配给数组元素的值是一个标量值,而不是一个序列。
阅读全文
相关推荐
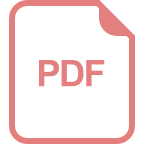
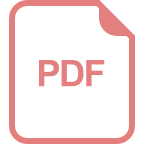
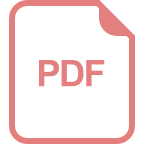








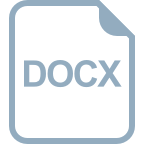
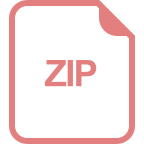