用c语言编写一个程序:藏文音节构件识别与统计。通过藏文音节构件识别算法,编程实现藏文构件识别,并对藏文每个构件字符实现统计 完整的程序
时间: 2024-03-27 22:36:20 浏览: 50
以下是一个简单的C语言程序,用于藏文音节构件识别与统计。这个程序可以从一个藏文文本文件中读取字符,并检测每个字符是否为一个藏文构件。如果是,程序将对该构件进行计数,并输出每个构件的统计结果。
```
#include <stdio.h>
#include <stdbool.h>
// 定义一个结构体来表示每个构件及其计数
struct Component {
char name[4];
int count;
};
int main() {
FILE *fp;
struct Component components[50]; // 假设最多有50个不同的构件
int num_components = 0;
// 打开输入文件
fp = fopen("input.txt", "r");
if (fp == NULL) {
printf("无法打开输入文件!\n");
return 1;
}
// 逐个字符读取文件内容
char c;
bool is_component = false;
char component_name[4];
while ((c = fgetc(fp)) != EOF) {
if (c >= 0xF0 && c <= 0xF3) {
// 如果这个字符是一个藏文构件的第一个字节,则开始记录构件名称
is_component = true;
component_name[0] = c;
} else if (is_component && c >= 0x80 && c <= 0xBF) {
// 如果这个字符是一个藏文构件的后续字节,则将其添加到构件名称中
component_name[1] = component_name[2];
component_name[2] = component_name[3];
component_name[3] = c;
if (component_name[3] != 0) {
// 如果已经读取了完整的四个字节,则将其添加到构件计数数组中
bool found = false;
for (int i = 0; i < num_components; i++) {
if (strcmp(components[i].name, component_name) == 0) {
components[i].count++;
found = true;
break;
}
}
if (!found) {
strcpy(components[num_components].name, component_name);
components[num_components].count = 1;
num_components++;
}
is_component = false;
memset(component_name, 0, sizeof(component_name));
}
} else {
// 如果这个字符不是一个藏文构件的字节,则将其视为一个新的构件
is_component = false;
memset(component_name, 0, sizeof(component_name));
}
}
// 关闭输入文件
fclose(fp);
// 输出每个构件的统计结果
for (int i = 0; i < num_components; i++) {
printf("%s: %d\n", components[i].name, components[i].count);
}
return 0;
}
```
请注意,这是一个非常基本的程序示例,仅供参考。如果您需要更复杂的功能,例如处理多个文件、支持不同的输入格式、提供更详细的统计信息等,则需要对程序进行更改和扩展。
阅读全文
相关推荐
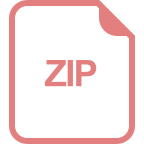
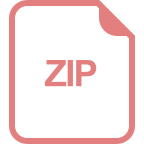
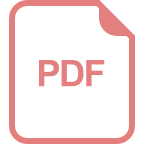
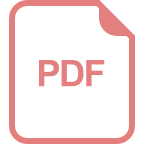
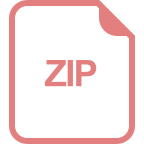
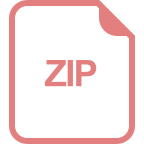
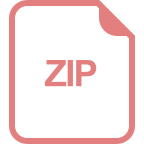
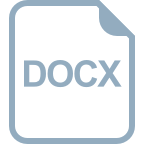
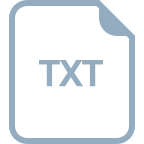