react native 在输入的时候键盘遮挡了输入框,如何解决
时间: 2023-11-28 09:49:26 浏览: 65
在 React Native 中,当键盘弹出时,会遮挡住输入框,这是一个常见的问题。你可以通过以下几种方式来解决:
1. 使用 KeyboardAvoidingView 组件
React Native 提供了 KeyboardAvoidingView 组件,可以自动调整页面的布局,以避免键盘遮挡输入框。你可以将输入框放在 KeyboardAvoidingView 组件中,然后设置 behavior 属性来控制布局的调整方式。例如:
```
import { KeyboardAvoidingView, TextInput } from 'react-native';
<KeyboardAvoidingView behavior={'padding'} style={{ flex: 1 }}>
<TextInput style={{ height: 40, borderColor: 'gray', borderWidth: 1 }} />
</KeyboardAvoidingView>
```
2. 使用 ScrollView 组件
如果页面上有多个输入框,你可以将它们放在一个 ScrollView 组件中,并在键盘弹出时滚动到当前输入框的位置上。你可以使用 scrollTo 方法来实现这个功能。例如:
```
import { ScrollView, TextInput } from 'react-native';
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
inputHeight: 40,
};
this.scrollView = React.createRef();
this.textInput = React.createRef();
}
componentDidMount() {
this.keyboardDidShowListener = Keyboard.addListener(
'keyboardDidShow',
this.keyboardDidShow
);
this.keyboardDidHideListener = Keyboard.addListener(
'keyboardDidHide',
this.keyboardDidHide
);
}
componentWillUnmount() {
this.keyboardDidShowListener.remove();
this.keyboardDidHideListener.remove();
}
keyboardDidShow = (event) => {
const keyboardHeight = event.endCoordinates.height;
const scrollResponder = this.scrollView.current.getScrollResponder();
const inputHandle = ReactNative.findNodeHandle(this.textInput.current);
const inputPosition = this.state.inputHeight + 10;
scrollResponder.scrollResponderScrollNativeHandleToKeyboard(
inputHandle,
inputPosition,
true
);
};
keyboardDidHide = () => {
const scrollResponder = this.scrollView.current.getScrollResponder();
scrollResponder.scrollResponderScrollTo({
x: 0,
y: 0,
animated: true,
});
};
render() {
return (
<ScrollView
ref={this.scrollView}
keyboardDismissMode="interactive"
contentContainerStyle={{ flexGrow: 1 }}
>
<TextInput
ref={this.textInput}
style={{ height: this.state.inputHeight }}
onFocus={() => this.setState({ inputHeight: 100 })}
onBlur={() => this.setState({ inputHeight: 40 })}
/>
<TextInput
ref={this.textInput}
style={{ height: this.state.inputHeight }}
onFocus={() => this.setState({ inputHeight: 100 })}
onBlur={() => this.setState({ inputHeight: 40 })}
/>
</ScrollView>
);
}
}
```
在上面的例子中,我们使用 ScrollView 组件包裹了两个 TextInput 组件,并且在 KeyboardDidShow 和 KeyboardDidHide 事件中,调用了 ScrollView 组件的 scrollTo 方法来滚动到当前输入框的位置上。
以上是两种比较常用的解决方法,你可以根据具体的场景选择适合自己的方式来解决问题。
阅读全文
相关推荐
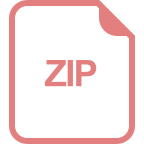
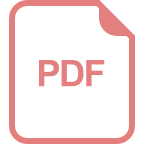
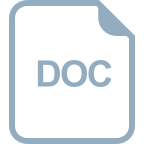
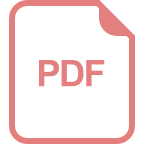
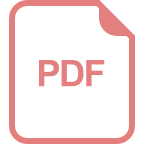
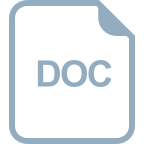
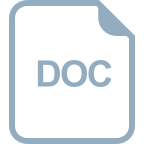
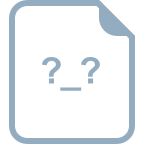
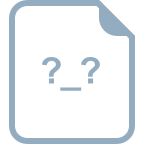
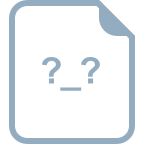
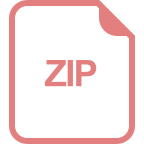
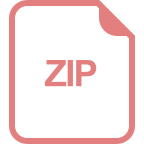
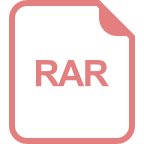
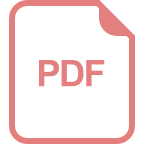
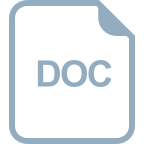
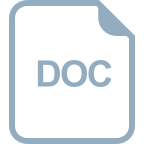
