C语言如何输入不确定行数的数据
时间: 2023-06-24 09:05:25 浏览: 294
在 C 语言中,可以使用循环结构来输入不确定行数的数据。常见的方法有两种:
1. 使用 while 循环
在 while 循环中,可以使用 scanf 函数来读入数据,每次读入一行,直到遇到文件结束符或特定的结束标记(比如输入-1)为止。示例代码如下:
```c
#include <stdio.h>
int main() {
int num;
while(scanf("%d", &num) != EOF && num != -1) {
printf("Input number: %d\n", num);
}
return 0;
}
```
2. 使用 do-while 循环
与 while 循环类似,do-while 循环也可以用来输入不确定行数的数据。不同的是,do-while 循环会先执行一次循环体,然后再进行条件判断。示例代码如下:
```c
#include <stdio.h>
int main() {
int num;
do {
printf("Input number: ");
scanf("%d", &num);
printf("You entered: %d\n", num);
} while(num != -1);
return 0;
}
```
无论使用哪种方法,都需要设置一个特定的结束标记,以便程序知道何时停止读入数据。
相关问题
如何用C语言按列读取数据
可以使用二维数组来按列读取数据。首先需要确定数据的行数和列数,然后使用循环读取每一列的数据并存储到二维数组中。以下是示例代码:
```
#include <stdio.h>
#define ROWS 3
#define COLS 4
int main() {
int data[ROWS][COLS];
int i, j;
// 读取数据
for (j = 0; j < COLS; j++) {
printf("请输入第 %d 列的数据:\n", j+1);
for (i = 0; i < ROWS; i++) {
scanf("%d", &data[i][j]);
}
}
// 输出数据
printf("读取的数据为:\n");
for (i = 0; i < ROWS; i++) {
for (j = 0; j < COLS; j++) {
printf("%d ", data[i][j]);
}
printf("\n");
}
return 0;
}
```
这段代码中,先定义了一个3行4列的二维数组data。然后使用循环依次读取每一列的数据,存储到data数组中。最后再循环输出data数组中的数据,即按列读取的结果。
用dem数据 c语言 填挖方算法
要使用DEM数据进行填挖方算法,需要先读取DEM数据文件,然后根据DEM数据计算每块土地的深度来确定是挖掉土方还是填方。下面是使用C语言实现填挖方算法并利用DEM数据的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int rows; // 行数
int cols; // 列数
float cellSize; // 栅格单元大小
float nodataValue; // 无效值
float *data; // DEM数据数组
} DEM;
// 读取DEM数据
void readDEM(char* fileName, DEM* dem)
{
FILE* fp = fopen(fileName, "rb");
if (fp == NULL)
{
printf("无法打开DEM数据文件 %s\n", fileName);
exit(1);
}
// 读取DEM文件头信息
fread(&(dem->cols), sizeof(int), 1, fp);
fread(&(dem->rows), sizeof(int), 1, fp);
fread(&(dem->cellSize), sizeof(float), 1, fp);
fread(&(dem->nodataValue), sizeof(float), 1, fp);
// 读取DEM数据
dem->data = (float*)malloc(sizeof(float) * dem->rows * dem->cols);
fread(dem->data, sizeof(float), dem->rows * dem->cols, fp);
fclose(fp);
}
// 计算每块土地的挖填方量
void calculateExcavationAndEmbankment(DEM* dem, float depth, float* excavation, float* embankment)
{
int i, j;
float V, sum = 0.0;
for (i = 0; i < dem->rows - 1; i++)
{
for (j = 0; j < dem->cols - 1; j++)
{
float z1 = dem->data[i * dem->cols + j];
float z2 = dem->data[i * dem->cols + j + 1];
float z3 = dem->data[(i + 1) * dem->cols + j];
float z4 = dem->data[(i + 1) * dem->cols + j + 1];
float zmin = z1;
if (z2 < zmin) zmin = z2;
if (z3 < zmin) zmin = z3;
if (z4 < zmin) zmin = z4;
float d = depth - zmin;
if (d > 0) // 深度为正表示挖掉了土方
{
V = dem->cellSize * dem->cellSize * d;
sum -= V;
}
else if (d < 0) // 深度为负表示填方
{
V = dem->cellSize * dem->cellSize * (-d);
sum += V;
}
}
}
*excavation = -sum;
*embankment = sum;
}
int main()
{
DEM dem;
float depth, excavation, embankment;
char fileName[256];
printf("请输入DEM数据文件名:");
scanf("%s", fileName);
readDEM(fileName, &dem);
printf("请输入深度:");
scanf("%f", &depth);
calculateExcavationAndEmbankment(&dem, depth, &excavation, &embankment);
printf("挖方量为:%.2f,填方量为:%.2f\n", excavation, embankment);
free(dem.data);
return 0;
}
```
该代码首先提示用户输入DEM数据文件名,然后读取DEM数据文件并将其存储在DEM结构体中。接下来提示用户输入深度,然后根据DEM数据计算每块土地的深度来确定是挖掉土方还是填方,并计算总的挖填方量。最后输出挖填方量并释放DEM数据数组所占用的内存。
相关推荐
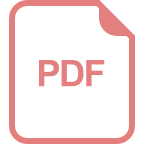












