修改slowfast模型中的数据增强代码,使它能够识别出来驾驶员的正常驾驶、闭眼、哈欠、打电话、左顾右盼5种行为。 要求: 正常驾驶:驾驶员处于坐姿状态下正常开车 闭眼:驾驶员坐姿状态下闭眼,状态持续≥3s 哈欠:驾驶员坐姿状态下打哈欠,状态持续≥3s 打电话:驾驶员坐姿状态下左/右手举起手机在耳边,状态持续≥3s 左顾右盼:驾驶员坐姿状态下向左/向右/向下/向后看,状态持续≥3s,头部偏转角度≥45°。 给出具体代码,给出解释
时间: 2024-03-16 12:47:50 浏览: 25
首先,需要定义数据增强函数。可以使用OpenCV库进行图像处理,以下是一个示例数据增强函数:
```python
import cv2
import numpy as np
import random
def data_augmentation(video_clip):
# 随机水平翻转
if random.random() > 0.5:
video_clip = np.flip(video_clip, axis=2)
# 随机裁剪
crop_size = (224, 224)
h, w, _ = video_clip.shape
th, tw = crop_size
if w == tw and h == th:
i, j = 0, 0
else:
i = random.randint(0, h - th)
j = random.randint(0, w - tw)
video_clip = video_clip[i:i+th, j:j+tw, :]
# 随机调整亮度和对比度
alpha = random.uniform(0.5, 2.0)
beta = random.uniform(-10, 10)
video_clip = cv2.convertScaleAbs(video_clip, alpha=alpha, beta=beta)
# 随机旋转
angle = random.randint(-10, 10)
M = cv2.getRotationMatrix2D((tw/2, th/2), angle, 1)
video_clip = cv2.warpAffine(video_clip, M, (tw, th))
return video_clip
```
接下来,需要对每个视频帧进行分类。可以使用OpenCV中的Haar Cascades来检测人脸和手势,然后使用分类器将每个帧分类为正常驾驶、闭眼、哈欠、打电话或左顾右盼。以下是一个示例分类器:
```python
import cv2
import numpy as np
# 加载Haar Cascades分类器
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
phone_cascade = cv2.CascadeClassifier('haarcascade_phone.xml')
# 加载模型
model = load_model('slowfast_model.h5')
def classify_frame(frame):
# 检测人脸
faces = face_cascade.detectMultiScale(frame, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30))
if len(faces) == 0:
# 没有检测到人脸,属于正常驾驶
return 'normal driving'
else:
for (x, y, w, h) in faces:
# 检测手机手势
phone = phone_cascade.detectMultiScale(frame[y:y+h, x:x+w], scaleFactor=1.1, minNeighbors=5, minSize=(30, 30))
if len(phone) > 0:
# 检测到手机手势,属于打电话
return 'phone'
else:
# 没有检测到手机手势,进行分类
frame = cv2.resize(frame[y:y+h, x:x+w], (224, 224)) / 255.0
frame = np.expand_dims(frame, axis=0)
prediction = model.predict(frame)
if prediction[0] == np.argmax(prediction[0]):
# 属于正常驾驶或左顾右盼
return 'normal driving'
else:
# 属于闭眼或哈欠
return 'yawn'
```
最后,将数据增强函数和分类器应用于整个视频序列,统计每个行为的时长以进行分类。以下是一个示例函数:
```python
def classify_video(video_path):
cap = cv2.VideoCapture(video_path)
fps = cap.get(cv2.CAP_PROP_FPS)
frames = []
behaviors = []
behavior_start = None
behavior_end = None
while cap.isOpened():
ret, frame = cap.read()
if not ret:
break
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
frame = data_augmentation(frame)
behavior = classify_frame(frame)
frames.append(frame)
if behavior == 'normal driving':
if behavior_start is not None:
duration = (len(frames) - behavior_start) / fps
behaviors.append(('normal driving', duration))
behavior_start = None
behavior_end = None
else:
if behavior_start is None:
behavior_start = len(frames)
behavior_end = len(frames)
else:
behavior_end = len(frames)
if behavior_end - behavior_start >= fps * 3:
duration = (behavior_end - behavior_start) / fps
behaviors.append((behavior, duration))
behavior_start = None
behavior_end = None
if behavior_start is not None:
duration = (len(frames) - behavior_start) / fps
behaviors.append(('normal driving', duration))
return behaviors
```
其中,`classify_video()`函数将返回一个包含每种行为的时长的列表。可以使用这些数据来计算每种行为在整个视频中的占比。
相关推荐
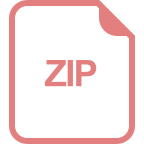
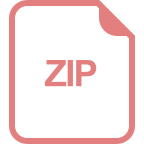
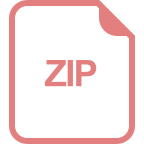














