vue3使用canvas或者svg,制作一个宽100px,高50px的心电图动态效果
时间: 2024-02-24 15:54:34 浏览: 137
好的,我可以为您提供相关的代码示例。
首先,我们需要在Vue3中创建一个组件,用于展示心电图动态效果。这个组件将使用Canvas来绘制。
```html
<template>
<canvas ref="canvas" :width="width" :height="height"></canvas>
</template>
```
在这个组件中,我们使用了`<canvas>`元素,并通过`ref`属性引用了它。我们还可以设置这个元素的宽度和高度。
接下来,我们需要在组件的JavaScript代码中编写绘制心电图的代码。我们可以使用`requestAnimationFrame()`函数来实现动态效果。
```javascript
<script>
export default {
data() {
return {
width: 100,
height: 50,
points: [],
lastTimestamp: 0
}
},
mounted() {
this.ctx = this.$refs.canvas.getContext('2d');
this.ctx.strokeStyle = 'red';
this.ctx.lineWidth = 1;
this.ctx.lineCap = 'round';
this.startAnimation();
},
methods: {
startAnimation() {
requestAnimationFrame(this.draw);
},
draw(timestamp) {
const dt = timestamp - this.lastTimestamp;
const x = this.width / 2;
const y = this.height / 2 + Math.sin(timestamp / 100) * 10;
this.points.push({ x, y });
this.ctx.clearRect(0, 0, this.width, this.height);
this.ctx.beginPath();
for (let i = 0; i < this.points.length; i++) {
const point = this.points[i];
if (i === 0) {
this.ctx.moveTo(point.x, point.y);
} else {
this.ctx.lineTo(point.x, point.y);
}
}
this.ctx.stroke();
this.points = this.points.filter(point => point.x > -10);
this.lastTimestamp = timestamp;
requestAnimationFrame(this.draw);
}
}
}
</script>
```
在这个代码中,我们首先在`mounted()`方法中获取了`<canvas>`元素的绘图上下文,并设置了一些绘图属性。然后,我们调用了`startAnimation()`方法,这个方法会在每一帧调用`draw()`方法来绘制心电图。
在`draw()`方法中,我们首先计算出当前时间与上一次绘制之间的时间差,然后计算出当前点的位置。我们将这个点添加到一个数组中,并绘制一条从数组中第一个点到最后一个点的线条。
为了实现动态效果,我们在每一帧中都清空画布,并将所有点向左移动一些距离。最后,我们需要调用`requestAnimationFrame()`函数来启动下一帧的绘制。
这个组件现在就可以在Vue3应用中使用了。只需要在需要的地方添加这个组件,就可以看到心电图动态效果了。
```html
<template>
<div>
<HeartRateChart />
</div>
</template>
<script>
import HeartRateChart from './components/HeartRateChart.vue'
export default {
components: {
HeartRateChart
}
}
</script>
```
阅读全文
相关推荐
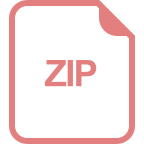
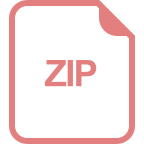
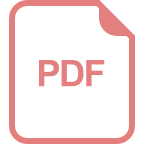
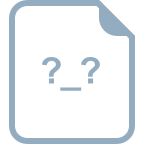
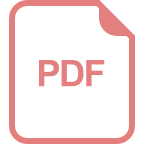
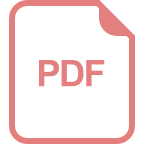
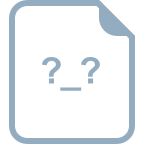
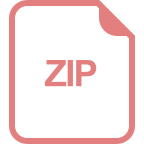
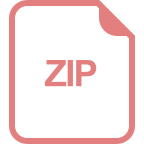
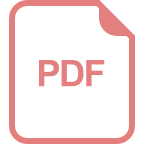
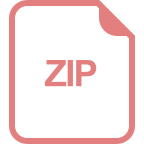
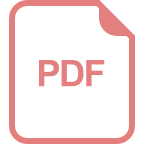
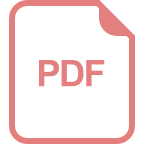