C#基于TCP/IP协议读写plc
时间: 2023-07-17 11:59:35 浏览: 82
在C#中,可以使用Socket类来实现基于TCP/IP协议的PLC通信。下面是一个简单的示例代码,演示如何通过TCP/IP协议读写PLC:
```csharp
using System;
using System.Net.Sockets;
class Program
{
static void Main(string[] args)
{
// PLC的IP地址和端口号
string plcIp = "192.168.0.1";
int plcPort = 502;
try
{
// 创建TCP客户端套接字
using (TcpClient client = new TcpClient())
{
// 连接到PLC
client.Connect(plcIp, plcPort);
// 获取网络流
using (NetworkStream stream = client.GetStream())
{
// 发送读取请求
byte[] readRequest = new byte[] { 0x00, 0x01, 0x00, 0x00, 0x00, 0x06, 0x01, 0x03, 0x00, 0x00, 0x00, 0x01 };
stream.Write(readRequest, 0, readRequest.Length);
// 接收响应数据
byte[] response = new byte[1024];
int bytesRead = stream.Read(response, 0, response.Length);
// 解析响应数据
// 这里根据PLC的协议进行解析,具体的解析方式需要参考PLC的文档
// 关闭连接
client.Close();
}
}
}
catch (Exception ex)
{
Console.WriteLine("PLC通信发生错误:" + ex.Message);
}
}
}
```
在上述示例中,我们使用TcpClient类来创建一个TCP客户端套接字,然后使用Connect方法连接到PLC的IP地址和端口号。接着,通过GetStream方法获取网络流,并通过Write方法发送读取请求。然后,通过Read方法接收响应数据,并根据PLC的协议进行解析。最后,关闭连接。
请注意,上述代码仅为示例,实际的PLC通信协议和数据解析方式可能因PLC型号和厂商而异。您需要参考PLC的文档以了解具体的通信协议和数据解析方式。
相关推荐
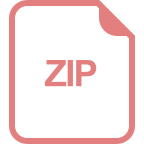
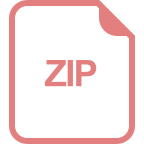
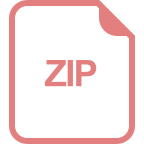
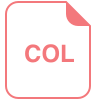
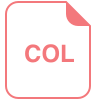












