C#实现PLC读写功能代码基于TCP代码
时间: 2024-01-08 19:03:06 浏览: 130
当使用C#实现PLC读写功能的代码基于TCP协议时,以下是一个示例代码:
```csharp
using System;
using System.Net.Sockets;
using System.Text;
public class PLCCommunication
{
private TcpClient client;
private NetworkStream stream;
private string plcIpAddress;
private int plcPort;
public PLCCommunication(string ipAddress, int port)
{
plcIpAddress = ipAddress;
plcPort = port;
}
public void Connect()
{
client = new TcpClient();
client.Connect(plcIpAddress, plcPort);
stream = client.GetStream();
}
public void Disconnect()
{
stream.Close();
client.Close();
}
public string ReadFromPLC(int address, int length)
{
// 构造读取命令
string command = "R" + address.ToString("X") + length.ToString("X") + "\r\n";
byte[] sendData = Encoding.ASCII.GetBytes(command);
// 发送命令
stream.Write(sendData, 0, sendData.Length);
// 接收数据
byte[] receiveData = new byte[1024];
int bytesRead = stream.Read(receiveData, 0, receiveData.Length);
string response = Encoding.ASCII.GetString(receiveData, 0, bytesRead);
return response;
}
public void WriteToPLC(int address, string data)
{
// 构造写入命令
string command = "W" + address.ToString("X") + data.Length.ToString("X") + data + "\r\n";
byte[] sendData = Encoding.ASCII.GetBytes(command);
// 发送命令
stream.Write(sendData, 0, sendData.Length);
}
}
```
使用上述代码的示例:
```csharp
static void Main()
{
PLCCommunication plc = new PLCCommunication("PLC_IP", PLC_Port);
try
{
plc.Connect();
// 读取PLC数据
string readData = plc.ReadFromPLC(0, 10);
Console.WriteLine("读取的数据:" + readData);
// 写入PLC数据
string writeData = "1234567890";
plc.WriteToPLC(0, writeData);
Console.WriteLine("写入成功!");
}
catch (Exception ex)
{
Console.WriteLine("发生异常:" + ex.Message);
}
finally
{
plc.Disconnect();
}
Console.ReadLine();
}
```
请注意,上述代码仅为示例,具体的实现细节可能因PLC型号、通信协议和要求的数据格式而有所不同。您需要根据您所使用的PLC和通信协议的要求进行相应的调整。
此外,代码中还可以添加错误处理和异常处理的逻辑,以增加程序的稳定性和可靠性。
希望这个示例代码对您有所帮助!如有任何进一步的问题,请随时提问。
阅读全文
相关推荐
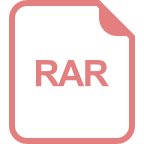
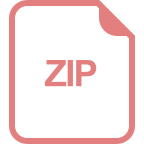
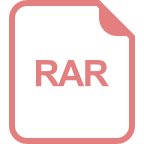
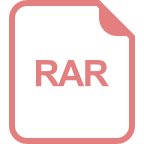
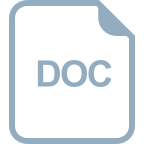
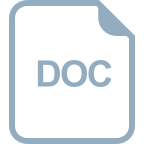
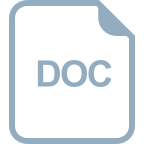



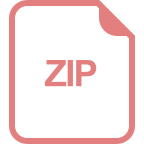
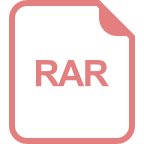
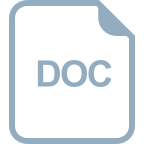
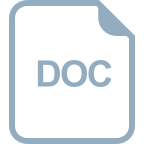
