org.springframework.web.bind.annotation.RequestBody
时间: 2023-09-17 13:06:27 浏览: 48
org.springframework.web.bind.annotation.RequestBody是Spring Framework中的注解之一,用于在处理HTTP请求时将请求体的内容绑定到方法参数上。通常情况下,我们使用POST、PUT、PATCH等请求方式向服务器提交数据时,都会将数据放在请求体中。使用@RequestBody注解可以将请求体中的数据自动转换为Java对象,从而方便我们进一步处理这些数据。例如:
```java
@PostMapping("/users")
public ResponseEntity<User> createUser(@RequestBody User user) {
// 处理用户创建逻辑
}
```
在上面的示例中,我们使用@RequestBody注解将请求体中的数据转换为一个User对象,并将其作为参数传递给createUser方法。Spring会自动根据请求体的内容来创建User对象,并将其绑定到方法参数上。这样,我们就可以方便地对用户提交的数据进行处理了。
相关问题
package com.example.teacher_admin_system.controller; import com.example.teacher_admin_system.pojo.Evaluation; import com.example.teacher_admin_system.pojo.JsonResult; import com.example.teacher_admin_system.service.EvaluationService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RestController; import java.util.List; @RestController public class EvaluationController { @Autowired private EvaluationService evaluationService; @GetMapping("/evaluation") public JsonResult<List<Evaluation>> findAll(){ return new JsonResult<>(200,"获取所有老师的平均分和评价数量",evaluationService.findAll()); } @GetMapping("/evaluation/{id}") public Evaluation findAll(@PathVariable("id") int id){ return evaluationService.findById(id); } @GetMapping("/evaluation/{name}") public Evaluation findAll(@PathVariable String name){ return evaluationService.findByName(name); } }检查其中的错误
该控制器代码存在两个问题:
1. 重载方法名相同
控制器中的两个方法名都是 "findAll",这会导致方法重载时出现问题。应该将第二个方法名改为 "findById" 或者其他不同的名字。
2. 参数类型不匹配
第三个方法中的参数应该是一个 path variable,而不是一个 request body。应该将 "@RequestBody" 改为 "@PathVariable"。
修改后的代码如下:
```java
package com.example.teacher_admin_system.controller;
import com.example.teacher_admin_system.pojo.Evaluation;
import com.example.teacher_admin_system.pojo.JsonResult;
import com.example.teacher_admin_system.service.EvaluationService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class EvaluationController {
@Autowired
private EvaluationService evaluationService;
@GetMapping("/evaluation")
public JsonResult<List<Evaluation>> findAll(){
return new JsonResult<>(200,"获取所有老师的平均分和评价数量",evaluationService.findAll());
}
@GetMapping("/evaluation/{id}")
public Evaluation findById(@PathVariable("id") int id){
return evaluationService.findById(id);
}
@GetMapping("/evaluation/name/{name}")
public Evaluation findByName(@PathVariable String name){
return evaluationService.findByName(name);
}
}
```
import org.springframework.beans.factory.annotation.Autowired;import org.springframework.http.ResponseEntity;import org.springframework.security.authentication.AuthenticationManager;import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;import org.springframework.security.core.Authentication;import org.springframework.security.core.userdetails.UserDetails;import org.springframework.web.bind.annotation.PostMapping;import org.springframework.web.bind.annotation.RequestBody;import org.springframework.web.bind.annotation.RestController;@RestControllerpublic class AuthController { @Autowired private AuthenticationManager authenticationManager; @Autowired private JwtTokenUtil jwtTokenUtil; @Autowired private UserDetailsService userDetailsService; @PostMapping("/login") public ResponseEntity<?> login(@RequestBody AuthRequest authRequest) throws Exception { try { Authentication authentication = authenticationManager.authenticate( new UsernamePasswordAuthenticationToken(authRequest.getUsername(), authRequest.getPassword()) ); UserDetails userDetails = userDetailsService.loadUserByUsername(authRequest.getUsername()); String token = jwtTokenUtil.generateToken(userDetails); return ResponseEntity.ok(new AuthResponse(token)); } catch (Exception e) { throw new Exception("Incorrect username or password", e); } }}中AuthRequest
是一个自定义类,用于接收用户登录请求中的用户名和密码。它可能长这样:
```
public class AuthRequest {
private String username;
private String password;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
```
在上面的代码中,我们定义了一个包含用户名和密码的类,可以通过setter和getter方法访问它们。这个类将被用作请求体来接收用户的登录请求。
相关推荐
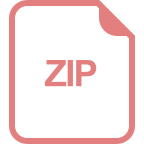
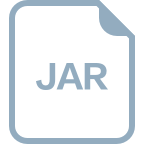












