java学生选课系统代码
时间: 2023-08-08 21:06:36 浏览: 53
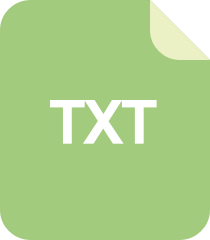
Java学生选课代码
这里提供一个简单的Java学生选课系统后端代码示例,基于Spring Boot框架实现:
1. 学生类:
```java
@Entity
@Table(name = "student")
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String password;
// 省略getter和setter方法
}
```
2. 课程类:
```java
@Entity
@Table(name = "course")
public class Course {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String teacher;
// 省略getter和setter方法
}
```
3. 选课类:
```java
@Entity
@Table(name = "selection")
public class Selection {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne
@JoinColumn(name = "student_id")
private Student student;
@ManyToOne
@JoinColumn(name = "course_id")
private Course course;
// 省略getter和setter方法
}
```
4. 学生服务类:
```java
@Service
public class StudentService {
@Autowired
private StudentRepository studentRepository;
public Student login(String name, String password) {
return studentRepository.findByNameAndPassword(name, password);
}
public List<Course> getCourses(Long studentId) {
return studentRepository.findById(studentId).get().getCourses();
}
public void selectCourse(Long studentId, Long courseId) {
Student student = studentRepository.findById(studentId).get();
Course course = courseRepository.findById(courseId).get();
Selection selection = new Selection();
selection.setStudent(student);
selection.setCourse(course);
selectionRepository.save(selection);
}
public void quitCourse(Long studentId, Long courseId) {
Student student = studentRepository.findById(studentId).get();
Course course = courseRepository.findById(courseId).get();
Selection selection = selectionRepository.findByStudentAndCourse(student, course);
if (selection != null) {
selectionRepository.delete(selection);
}
}
}
```
5. 控制器类:
```java
@RestController
@RequestMapping("/api/student")
public class StudentController {
@Autowired
private StudentService studentService;
@PostMapping("/login")
public Student login(@RequestParam String name, @RequestParam String password) {
return studentService.login(name, password);
}
@GetMapping("/courses")
public List<Course> getCourses(@RequestParam Long studentId) {
return studentService.getCourses(studentId);
}
@PostMapping("/selectCourse")
public void selectCourse(@RequestParam Long studentId, @RequestParam Long courseId) {
studentService.selectCourse(studentId, courseId);
}
@PostMapping("/quitCourse")
public void quitCourse(@RequestParam Long studentId, @RequestParam Long courseId) {
studentService.quitCourse(studentId, courseId);
}
}
```
以上代码仅为示例,具体实现需要根据实际需求进行调整和优化。
阅读全文
相关推荐
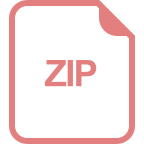
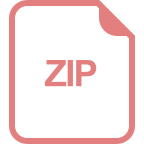
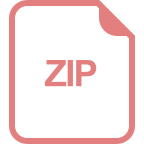
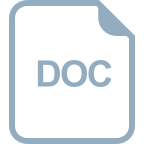
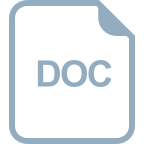
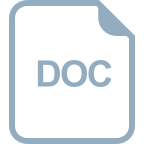
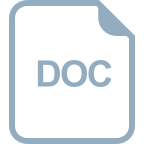
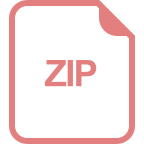
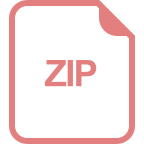


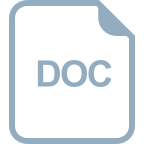