用C语言编写一个程序,使之接受C源码文件路径作为运行参数,读取该文件中内容,对于所遇到的#include<...>和#include"..."预处理指令,将该导入指令替换为对应头文件的具体内容文本,并将执行替换后的整体内容文本输出到第二个运行参数表示的文件位置中。
时间: 2024-02-22 19:58:55 浏览: 162
好的,我会尽力回答您的问题。
以下是用C语言编写的程序,可以实现您的需求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_PATH_LENGTH 256
#define MAX_FILE_LENGTH 1024
void replace_include(char* source_path, char* dest_path) {
FILE* source_file = fopen(source_path, "r");
FILE* dest_file = fopen(dest_path, "w");
if (source_file == NULL) {
fprintf(stderr, "Error: Failed to open source file.\n");
exit(1);
}
if (dest_file == NULL) {
fprintf(stderr, "Error: Failed to open destination file.\n");
exit(1);
}
char line[MAX_FILE_LENGTH];
while (fgets(line, MAX_FILE_LENGTH, source_file)) {
if (strncmp(line, "#include", strlen("#include")) == 0) {
char* start = strchr(line, '<');
char* end = strchr(line, '>');
if (start != NULL && end != NULL) {
char header_path[MAX_PATH_LENGTH];
strncpy(header_path, start + 1, end - start - 1);
header_path[end - start - 1] = '\0';
FILE* header_file = fopen(header_path, "r");
if (header_file == NULL) {
fprintf(stderr, "Error: Failed to open included header file.\n");
exit(1);
}
char header_line[MAX_FILE_LENGTH];
while (fgets(header_line, MAX_FILE_LENGTH, header_file)) {
fprintf(dest_file, "%s", header_line);
}
fclose(header_file);
} else {
start = strchr(line, '\"');
end = strrchr(line, '\"');
if (start != NULL && end != NULL) {
char header_path[MAX_PATH_LENGTH];
strncpy(header_path, start + 1, end - start - 1);
header_path[end - start - 1] = '\0';
FILE* header_file = fopen(header_path, "r");
if (header_file == NULL) {
fprintf(stderr, "Error: Failed to open included header file.\n");
exit(1);
}
char header_line[MAX_FILE_LENGTH];
while (fgets(header_line, MAX_FILE_LENGTH, header_file)) {
fprintf(dest_file, "%s", header_line);
}
fclose(header_file);
}
}
} else {
fprintf(dest_file, "%s", line);
}
}
fclose(source_file);
fclose(dest_file);
}
int main(int argc, char* argv[]) {
if (argc < 3) {
fprintf(stderr, "Usage: %s <source_file_path> <dest_file_path>\n", argv[0]);
exit(1);
}
char* source_path = argv[1];
char* dest_path = argv[2];
replace_include(source_path, dest_path);
return 0;
}
```
该程序的主要思路是读取源文件,逐行扫描文件内容,对于每一行中包含的#include<...>和#include"..."预处理指令,读取对应的头文件内容并插入到目标文件中,最终将替换后的整体内容写入到目标文件中。需要注意的是,程序中的一些常量值是根据实际情况设定的,您需要根据自己的需求进行修改。
希望我的回答对您有所帮助!
阅读全文
相关推荐
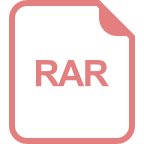
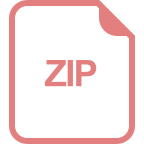
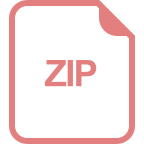















