C语言链式队列打印杨辉三角代码
时间: 2023-11-12 09:03:52 浏览: 87
当然可以,我帮你查找一下 C 语言链式队列打印杨辉三角的代码:
```
#include <stdio.h>
#include <stdlib.h>
// 链式队列节点
typedef struct QNode {
int data;
struct QNode *next;
} QNode, *QueuePtr;
// 链式队列结构体
typedef struct {
QueuePtr front; // 队头指针
QueuePtr rear; // 队尾指针
} LinkQueue;
// 初始化队列
void InitQueue(LinkQueue *Q)
{
Q->front = Q->rear = (QueuePtr)malloc(sizeof(QNode));
if (!Q->front) {
exit(-1);
}
Q->front->next = NULL;
}
// 入队列
void EnQueue(LinkQueue *Q, int e)
{
QueuePtr p = (QueuePtr)malloc(sizeof(QNode));
if (!p) {
exit(-1);
}
p->data = e;
p->next = NULL;
Q->rear->next = p;
Q->rear = p;
}
// 出队列
int DeQueue(LinkQueue *Q)
{
int e;
QueuePtr p;
if (Q->front == Q->rear) {
return -1;
}
p = Q->front->next;
e = p->data;
Q->front->next = p->next;
if (Q->rear == p) {
Q->rear = Q->front;
}
free(p);
return e;
}
// 返回队列长度
int GetQueueLength(LinkQueue *Q)
{
int len = 0;
QueuePtr p = Q->front->next;
while (p) {
len++;
p = p->next;
}
return len;
}
// 打印杨辉三角,num 表示打印多少行
void print_yh_triangle(int num)
{
int i, j, a[num];
LinkQueue Q;
InitQueue(&Q);
for (i = 0; i < num; i++) {
for (j = 0; j <= i; j++) {
if (j == 0 || j == i) {
a[j] = 1;
} else {
a[j] = DeQueue(&Q) + DeQueue(&Q);// 取出队列的前两个元素相加,组成新的元素入队列
}
printf("%d ", a[j]);
EnQueue(&Q, a[j]);// 新元素入队列
}
printf("\n");
}
}
int main()
{
print_yh_triangle(5);
return 0;
}
```
希望这个代码对你有所帮助。
阅读全文
相关推荐
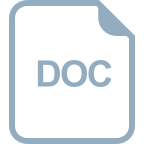



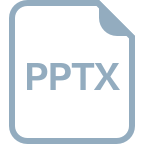
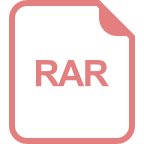
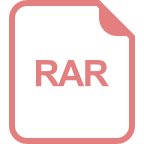
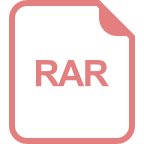
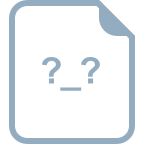
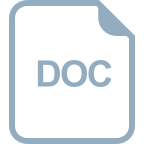
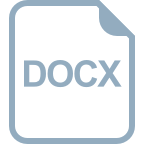
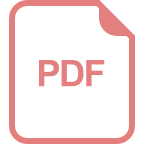
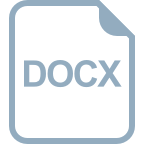
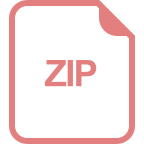