请帮我用keras编写一个简单的全连接神经网络,用来训练一组简单的任意散点,是回归问题,在神经网络中加入pso算法对超参数进行优化,要优化的超参数包括隐藏层层数,每层神经元个数,学习率。
时间: 2024-05-12 08:20:42 浏览: 94
下面是一个简单的全连接神经网络,使用Keras编写,用于训练一组简单的任意散点。同时,我们使用PSO算法对超参数进行优化,包括隐藏层层数,每层神经元个数和学习率。
```python
import numpy as np
from keras.models import Sequential
from keras.layers import Dense
from keras.optimizers import Adam
from pyswarm import pso
# Generate some random data
x = np.random.rand(100, 2)
y = np.sum(x, axis=1)
# Define the model
def create_model(n_layers, n_neurons, learning_rate):
model = Sequential()
model.add(Dense(n_neurons, input_dim=2, activation='relu'))
for i in range(n_layers-1):
model.add(Dense(n_neurons, activation='relu'))
model.add(Dense(1, activation='linear'))
optimizer = Adam(lr=learning_rate)
model.compile(loss='mean_squared_error', optimizer=optimizer)
return model
# Define the objective function to be optimized by PSO
def objective_function(x):
n_layers = int(x[0])
n_neurons = int(x[1])
learning_rate = 10**x[2]
model = create_model(n_layers, n_neurons, learning_rate)
history = model.fit(x, y, epochs=10, verbose=0)
loss = history.history['loss'][-1]
return loss
# Define the bounds for the PSO algorithm
lb = [1, 1, -5]
ub = [10, 100, 0]
# Use PSO to optimize the hyperparameters
xopt, fopt = pso(objective_function, lb, ub, swarmsize=10, maxiter=50)
# Train the final model with the optimized hyperparameters
n_layers = int(xopt[0])
n_neurons = int(xopt[1])
learning_rate = 10**xopt[2]
final_model = create_model(n_layers, n_neurons, learning_rate)
final_model.fit(x, y, epochs=100, verbose=0)
```
在上面的代码中,我们首先生成了一组随机数据 `x` 和 `y`,其中 `x` 是一个 $100\times2$ 的矩阵,`y` 是一个长度为 100 的向量。接着,我们定义了一个函数 `create_model`,该函数用于创建一个全连接神经网络模型,并使用给定的超参数配置对其进行编译。其中,隐藏层的层数和神经元数量由 `n_layers` 和 `n_neurons` 控制,学习率由 `learning_rate` 控制。
然后,我们定义了一个函数 `objective_function`,该函数用于计算模型在给定超参数下的损失。我们使用该函数作为 PSO 算法的目标函数来优化超参数。最后,我们使用 `pso` 函数从给定的上下界范围内寻找最优超参数,并使用最优超参数训练最终的模型。
需要注意的是,上述代码仅仅是一个示例,实际使用时需要根据具体问题进行修改和优化。例如,可以考虑使用交叉验证来评估模型的性能,或者使用更复杂的网络结构和正则化方法来提高模型的泛化能力。
阅读全文
相关推荐
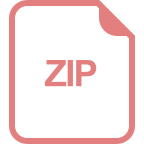
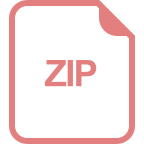
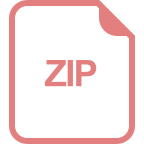
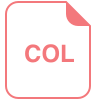
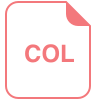
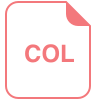
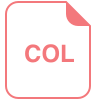

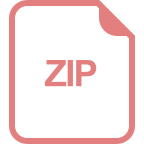
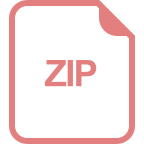
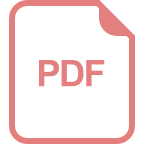
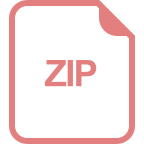
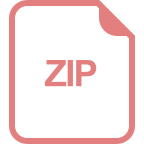