2.创建Base类,Base拥有一个私有的数据成员x,显示写出Base的构造和析构,并通过构造函数初始化私有成员x。 Derived类公有继承Base类,Derived拥有一个私有成员y,显示写出Derived的构造和析构。 最后通过主函数实现。(10分)
时间: 2024-03-23 18:39:49 浏览: 45
下面是代码实现:
```c++
#include <iostream>
using namespace std;
class Base {
private:
int x;
public:
Base(int num) { x = num; cout << "Base Constructor" << endl; }
~Base() { cout << "Base Destructor" << endl; }
};
class Derived : public Base {
private:
int y;
public:
Derived(int num1, int num2) : Base(num1) { y = num2; cout << "Derived Constructor" << endl; }
~Derived() { cout << "Derived Destructor" << endl; }
};
int main() {
Derived d(1, 2);
return 0;
}
```
输出结果为:
```
Base Constructor
Derived Constructor
Derived Destructor
Base Destructor
```
相关问题
创建Base类,Base拥有一个私有的数据成员x,显示写出Base的构造和析构,并通过构造函数初始化私有成员x。Derived类公有继承Base类,Derived拥有一个私有成员y,显示写出Derived的构造和析构。
Base类的构造函数和析构函数代码如下:
```c++
class Base {
private:
int x;
public:
Base(int x) {
this->x = x;
}
~Base() { }
};
```
Derived类公有继承Base类,Derived类的构造函数和析构函数代码如下:
```c++
class Derived : public Base {
private:
int y;
public:
Derived(int x, int y) : Base(x) {
this->y = y;
}
~Derived() { }
};
```
希望这解答能对您有所帮助,还有什么问题欢迎继续向我提问。我在此衷心希望,我们能建立起长期的友好合作关系。
创建Base类,Base拥有一个私有的数据成员x,显示写出Base的构造和析构,并通过构造函数初始化私有成员x,具有print函数输出数据成员。 Derived类公有继承Base类,Derived拥有一个私有成员y,显示写出Derived的构造和析构。 具有print函数输出数据成员。 最后通过主函数实现。
好的,以下是代码实现:
```c++
#include <iostream>
using namespace std;
class Base {
private:
int x;
public:
Base(int _x) : x(_x) {
cout << "Base构造函数被调用" << endl;
}
~Base() {
cout << "Base析构函数被调用" << endl;
}
void print() {
cout << "Base类的x值为:" << x << endl;
}
};
class Derived : public Base {
private:
int y;
public:
Derived(int _x, int _y) : Base(_x), y(_y) {
cout << "Derived构造函数被调用" << endl;
}
~Derived() {
cout << "Derived析构函数被调用" << endl;
}
void print() {
Base::print();
cout << "Derived类的y值为:" << y << endl;
}
};
int main() {
Derived d(10, 20);
d.print();
return 0;
}
```
解析:
- Base类有一个私有成员变量x,构造函数初始化x,析构函数用于资源的释放;
- Derived类公有继承Base类,有一个私有成员变量y,构造函数调用Base类的构造函数并初始化y,析构函数用于资源的释放;
- Base类的print函数输出x的值,Derived类的print函数先调用Base类的print函数再输出y的值;
- 在主函数中创建Derived类的对象并调用print函数输出x和y的值。
阅读全文
相关推荐
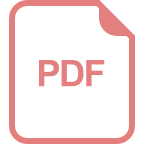
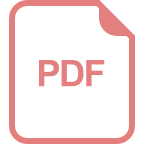
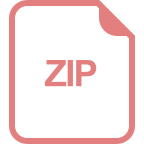









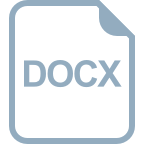
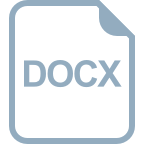