编写一个java程序求解迷宫问题。要求输出迷宫并输出是否有通路,并以适当的形式输出路径。
时间: 2024-11-29 18:37:07 浏览: 13
编写一个Java程序解决迷宫问题通常会涉及深度优先搜索(Depth First Search, DFS)或广度优先搜索(Breadth First Search, BFS)。这里我会给出一个简单的DFS解决方案作为示例:
```java
import java.util.*;
public class MazeSolver {
private char[][] maze; // 迷宫矩阵,0表示空地,1表示墙壁
private int startRow, startCol, endRow, endCol;
public MazeSolver(char[][] maze, int startRow, int startCol, int endRow, int endCol) {
this.maze = maze;
this.startRow = startRow;
this.startCol = startCol;
this.endRow = endCol;
this.endCol = endCol;
}
// 检查是否可达
public boolean isReachable() {
return dfs(startRow, startCol);
}
// DFS遍历算法
private boolean dfs(int row, int col) {
if (row < 0 || row >= maze.length || col < 0 || col >= maze[0].length || maze[row][col] == '1') {
return false; // 遇到墙壁或者越界
}
if (row == endRow && col == endCol) {
System.out.println("找到了一条路径:");
printPath(row, col);
return true;
}
maze[row][col] = '1'; // 标记已访问过
if (dfs(row + 1, col) || dfs(row - 1, col) || dfs(row, col + 1) || dfs(row, col - 1)) {
System.out.print(maze[row][col] + " ");
return true;
}
maze[row][col] = '0'; // 如果回溯,恢复原状态
return false;
}
// 打印路径
private void printPath(int row, int col) {
Stack<int[]> stack = new Stack<>();
stack.push(new int[]{row, col});
while (!stack.isEmpty()) {
int[] currentPos = stack.pop();
System.out.print("(" + currentPos[0] + ", " + currentPos[1] + ")");
if (currentPos[0] != startRow || currentPos[1] != startCol) {
System.out.print(" -> ");
}
}
System.out.println("\n");
}
public static void main(String[] args) {
char[][] maze = { {'0', '1', '0', '1'},
{'1', '0', '0', '0'},
{'1', '1', '1', '1'},
{'0', '0', '1', '0'} };
MazeSolver solver = new MazeSolver(maze, 0, 0, 3, 3);
if (solver.isReachable()) {
System.out.println("迷宫有通路并且路径如下:");
} else {
System.out.println("迷宫不通路.");
}
}
}
```
在这个例子中,我们首先定义了一个`MazeSolver`类,它接受迷宫矩阵、起始位置和结束位置作为参数。`isReachable()`函数调用`dfs`方法检查从起点到终点是否存在通路。如果找到路径,它将打印出路径信息。如果没有通路,则输出提示。
运行这个程序,你将得到迷宫的状态以及是否能找到通路及其路径。
阅读全文
相关推荐
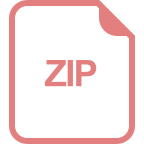
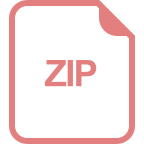
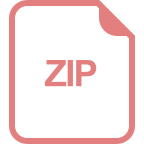





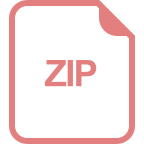
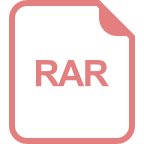