用c语言写一个循环生成多个单链表的函数,并把每个单链表的头节点指针存放在一个指针数组中
时间: 2023-03-28 14:04:55 浏览: 90
可以的,以下是一个示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#define LIST_SIZE 5
typedef struct Node {
int data;
struct Node *next;
} Node;
Node *createList(int size) {
Node *head = NULL;
Node *tail = NULL;
for (int i = ; i < size; i++) {
Node *node = (Node *)malloc(sizeof(Node));
node->data = i;
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
void createLists(Node **lists, int size) {
for (int i = ; i < size; i++) {
lists[i] = createList(i + 1);
}
}
int main() {
Node *lists[LIST_SIZE];
createLists(lists, LIST_SIZE);
for (int i = ; i < LIST_SIZE; i++) {
Node *head = lists[i];
printf("List %d: ", i);
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
return ;
}
```
这个程序定义了一个 `Node` 结构体表示单链表的节点,其中包含一个整数 `data` 和一个指向下一个节点的指针 `next`。`createList` 函数可以创建一个指定大小的单链表,并返回头节点的指针。`createLists` 函数可以循环调用 `createList` 函数来生成多个单链表,并将它们的头节点指针存放在一个指针数组中。最后,程序循环遍历这个指针数组,输出每个单链表的内容。
阅读全文
相关推荐
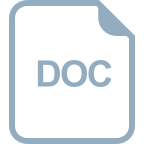
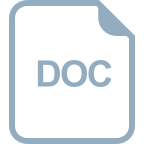
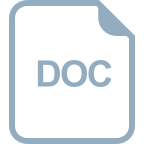
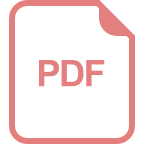
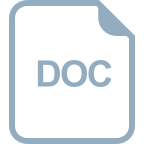
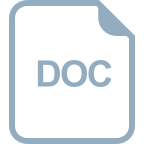











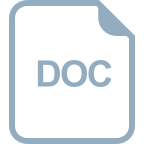
