C语言 单链表传递函数指针
时间: 2023-10-19 19:08:03 浏览: 202
你可以使用函数指针传递单链表,在C语言中,函数指针可以作为参数传递给其他函数,让函数能够调用指定的函数。
下面是一个示例代码,展示了如何使用函数指针传递单链表:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义单链表节点结构体
struct Node {
int data;
struct Node* next;
};
// 定义打印节点数据的函数
void printData(struct Node* node) {
printf("%d ", node->data);
}
// 定义遍历链表的函数,接受一个函数指针作为参数
void traverse(struct Node* head, void (*func)(struct Node*)) {
struct Node* current = head;
while (current != NULL) {
// 调用传入的函数指针
func(current);
current = current->next;
}
}
int main() {
// 创建链表
struct Node* head = (struct Node*)malloc(sizeof(struct Node));
struct Node* second = (struct Node*)malloc(sizeof(struct Node));
struct Node* third = (struct Node*)malloc(sizeof(struct Node));
head->data = 1;
head->next = second;
second->data = 2;
second->next = third;
third->data = 3;
third->next = NULL;
// 使用函数指针遍历并打印链表数据
traverse(head, printData);
// 释放内存
free(head);
free(second);
free(third);
return 0;
}
```
在上述代码中,我们定义了一个 `printData` 函数来打印节点的数据,然后我们定义了一个 `traverse` 函数,该函数接受一个指向 `printData` 函数的函数指针作为参数。在 `traverse` 函数内部,我们通过调用传入的函数指针来打印节点的数据。
在 `main` 函数中,我们创建了一个包含三个节点的单链表,并通过调用 `traverse` 函数来遍历并打印链表的数据。
注意:在使用函数指针传递链表时,确保函数指针与链表的节点类型匹配,以正确访问和操作链表的数据。
阅读全文
相关推荐
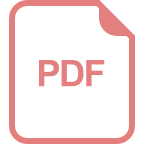
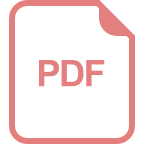
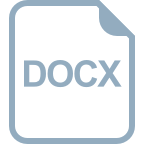















