建立二叉树,并实现二叉树的遍历,先序遍历采用递归算法实现,层次遍历用非递归算法来实现。
时间: 2024-03-09 09:47:20 浏览: 91
好的,我来为您解答这个问题。
首先,我们需要了解二叉树的基本概念。二叉树是由节点组成的树形结构,每个节点最多有两个子节点,分别称为左子节点和右子节点。二叉树有三种基本遍历方式:
- 先序遍历:先访问根节点,然后访问左子树,最后访问右子树。
- 中序遍历:先访问左子树,然后访问根节点,最后访问右子树。
- 后序遍历:先访问左子树,然后访问右子树,最后访问根节点。
现在我们来看一下如何建立二叉树和实现二叉树的先序遍历和层次遍历。
1. 建立二叉树
我们可以通过递归的方式来建立二叉树。具体实现如下:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def build_tree(preorder, inorder):
if not preorder or not inorder:
return None
root_val = preorder.pop(0)
root_index = inorder.index(root_val)
root = TreeNode(root_val)
root.left = build_tree(preorder, inorder[:root_index])
root.right = build_tree(preorder, inorder[root_index+1:])
return root
```
这里我们假设已知先序遍历结果和中序遍历结果,通过递归的方式建立二叉树。具体过程如下:
- 先序遍历的第一个元素是根节点,我们取出它的值,然后在中序遍历中找到该值的位置,这样就可以确定左子树和右子树的节点个数。
- 通过递归的方式分别构建左子树和右子树,然后将它们连接到根节点上。
2. 实现先序遍历
二叉树的先序遍历可以采用递归算法实现,具体实现如下:
```python
def preorder_traversal(root):
if not root:
return []
res = [root.val]
res += preorder_traversal(root.left)
res += preorder_traversal(root.right)
return res
```
这里我们先访问根节点,然后递归访问左子树和右子树,并将它们的结果合并起来。
3. 实现层次遍历
二叉树的层次遍历可以采用队列的方式实现,具体实现如下:
```python
from collections import deque
def level_traversal(root):
if not root:
return []
res = []
queue = deque([root])
while queue:
level_size = len(queue)
level_res = []
for i in range(level_size):
node = queue.popleft()
level_res.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
res.append(level_res)
return res
```
这里我们使用队列来存储每一层的节点,并逐层遍历。具体过程如下:
- 首先将根节点入队。
- 每次取出队列中的所有节点,将它们的值存储到一个列表中,并将它们的左右子节点入队。
- 将每一层的节点值存储到一个列表中,并将该列表存储到结果列表中。
这样就可以实现二叉树的层次遍历了。
以上就是建立二叉树,并实现二叉树的遍历的方法,希望对您有所帮助。
阅读全文
相关推荐
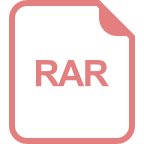
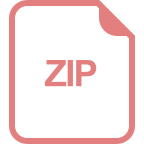













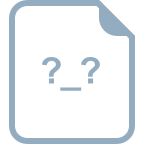