linux自旋锁测试脚本
时间: 2023-10-28 12:57:17 浏览: 37
以下是一个简单的 Linux 自旋锁测试脚本,它包含两个线程,一个线程获取锁并保持 5 秒钟,另一个线程在此期间尝试获取锁。脚本使用 shell 和 C 编写,需要安装 gcc 和 make。
```bash
#!/bin/bash
# Compile the C program
make spinlock
# Run the program with two threads
./spinlock 2 &
# Wait for the program to finish
wait
# Clean up
make clean
```
下面是 C 代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
pthread_spinlock_t lock;
void *thread_func(void *arg) {
int id = *(int *) arg;
int i;
// Try to acquire the lock
for (i = 0; i < 5; i++) {
printf("Thread %d trying to acquire lock\n", id);
pthread_spin_lock(&lock);
printf("Thread %d acquired lock\n", id);
sleep(1);
pthread_spin_unlock(&lock);
printf("Thread %d released lock\n", id);
sleep(1);
}
return NULL;
}
int main(int argc, char *argv[]) {
int num_threads = atoi(argv[1]);
int i;
// Initialize the spinlock
pthread_spin_init(&lock, 0);
// Create threads
pthread_t threads[num_threads];
int thread_ids[num_threads];
for (i = 0; i < num_threads; i++) {
thread_ids[i] = i + 1;
pthread_create(&threads[i], NULL, &thread_func, &thread_ids[i]);
}
// Wait for threads to finish
for (i = 0; i < num_threads; i++) {
pthread_join(threads[i], NULL);
}
// Destroy the spinlock
pthread_spin_destroy(&lock);
return 0;
}
```
该程序使用 pthread 库中的自旋锁。在主函数中,它创建指定数量的线程,并将每个线程的 ID 传递给线程函数。线程函数尝试获取锁并保持 5 秒钟,然后释放锁。在 shell 脚本中,它使用 make 编译 C 代码并运行程序,最后清理生成的文件。
相关推荐
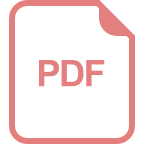
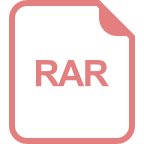
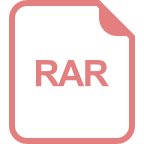














