在TK界面程序中添加一个”自动截图“的按钮和”停止截图“按钮,点击“自动截图”后每5秒自动调用截图函数,点击“停止截图”后停止调用截图函数
时间: 2023-11-10 16:05:24 浏览: 60
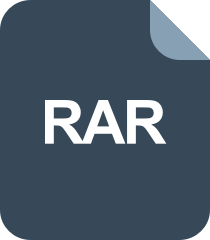
这是一个每隔5秒自动截屏一次的程序

实现该功能需要使用Python的`tkinter`和`PIL`库。
首先,我们需要创建一个基本的界面框架,包含“自动截图”和“停止截图”两个按钮。
```python
import tkinter as tk
class Application(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.pack()
self.create_widgets()
def create_widgets(self):
self.auto_screenshot_button = tk.Button(self)
self.auto_screenshot_button["text"] = "自动截图"
self.auto_screenshot_button["command"] = self.auto_screenshot
self.auto_screenshot_button.pack(side="left")
self.stop_screenshot_button = tk.Button(self)
self.stop_screenshot_button["text"] = "停止截图"
self.stop_screenshot_button["command"] = self.stop_screenshot
self.stop_screenshot_button.pack(side="left")
def auto_screenshot(self):
pass
def stop_screenshot(self):
pass
root = tk.Tk()
app = Application(master=root)
app.mainloop()
```
现在,我们需要实现`auto_screenshot`和`stop_screenshot`函数。`auto_screenshot`函数需要每5秒自动截图,并将截图保存到指定的文件夹中。我们可以使用`PIL`库的`ImageGrab`模块来实现截图功能。同时,我们需要在`auto_screenshot`函数中创建一个定时器,以实现每5秒截图的功能。当用户点击“停止截图”按钮时,我们需要停止该定时器。
```python
from PIL import ImageGrab
import time
import threading
class Application(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.pack()
self.create_widgets()
self.is_screenshotting = False
def create_widgets(self):
self.auto_screenshot_button = tk.Button(self)
self.auto_screenshot_button["text"] = "自动截图"
self.auto_screenshot_button["command"] = self.auto_screenshot
self.auto_screenshot_button.pack(side="left")
self.stop_screenshot_button = tk.Button(self)
self.stop_screenshot_button["text"] = "停止截图"
self.stop_screenshot_button["command"] = self.stop_screenshot
self.stop_screenshot_button.pack(side="left")
def auto_screenshot(self):
if not self.is_screenshotting:
self.is_screenshotting = True
self.screenshot_thread = threading.Thread(target=self.screenshot_loop)
self.screenshot_thread.start()
def stop_screenshot(self):
if self.is_screenshotting:
self.is_screenshotting = False
def screenshot_loop(self):
while self.is_screenshotting:
# 截图
im = ImageGrab.grab()
# 保存截图
im.save("screenshot_{}.png".format(time.time()))
# 等待5秒
time.sleep(5)
root = tk.Tk()
app = Application(master=root)
app.mainloop()
```
在这个版本的代码中,我们使用了一个`is_screenshotting`变量来记录当前是否正在截图。当用户点击“自动截图”按钮时,我们创建一个新的线程来执行`self.screenshot_loop`函数,该函数实际上就是一个死循环,每次循环中都会执行截图和保存截图的操作,并等待5秒。当用户点击“停止截图”按钮时,我们将`is_screenshotting`变量设置为`False`,从而停止循环。注意,在`auto_screenshot`函数中,我们需要检查`is_screenshotting`变量,以避免创建多个线程。
阅读全文
相关推荐
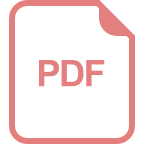
















