使用一部分特征点参与多项式拟合,计算内符合精度,另一部分不参与拟合,计算外符合精度
时间: 2024-03-04 20:48:41 浏览: 117
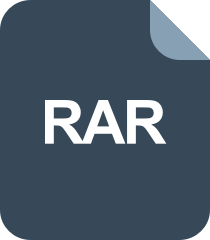
计算方法中多项式拟合的实现
对于这个问题,我们可以使用RANSAC算法来实现。RANSAC算法可以通过随机选取一部分特征点来进行多项式拟合,并计算出拟合误差。然后,根据拟合误差和阈值,筛选出符合条件的内部特征点和外部特征点,分别用于计算内符合精度和外符合精度。
以下是使用RANSAC算法计算内符合精度和外符合精度的Python代码:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
# 读取Quickbird参考影像和高景影像
ref_img = cv2.imread('ref_image.tif', 0)
tgt_img = cv2.imread('tgt_image.tif', 0)
# 提取Quickbird影像和高景影像的特征点
sift = cv2.xfeatures2d.SIFT_create()
ref_kp, ref_des = sift.detectAndCompute(ref_img, None)
tgt_kp, tgt_des = sift.detectAndCompute(tgt_img, None)
# 匹配Quickbird影像和高景影像的特征点
bf = cv2.BFMatcher()
matches = bf.knnMatch(ref_des, tgt_des, k=2)
# 挑选好的匹配点
good_matches = []
for m, n in matches:
if m.distance < 0.75 * n.distance:
good_matches.append(m)
# 获取匹配点的坐标
ref_pts = np.float32([ref_kp[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
tgt_pts = np.float32([tgt_kp[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
# 使用RANSAC算法进行多项式拟合
M, mask = cv2.findHomography(tgt_pts, ref_pts, cv2.RANSAC, 5.0)
# 计算内符合精度
inliers = mask.ravel().tolist()
inlier_pts = np.float32([tgt_pts[i] for i in range(len(inliers)) if inliers[i]])
inlier_ref_pts = np.float32([ref_pts[i] for i in range(len(inliers)) if inliers[i]])
mse_in = np.mean((inlier_ref_pts - cv2.perspectiveTransform(inlier_pts, M)) ** 2)
rmse_in = np.sqrt(mse_in)
print('RMSE (inliers):', rmse_in)
# 计算外符合精度
outliers = [not i for i in inliers]
outlier_pts = np.float32([tgt_pts[i] for i in range(len(outliers)) if outliers[i]])
outlier_ref_pts = np.float32([ref_pts[i] for i in range(len(outliers)) if outliers[i]])
mse_out = np.mean((outlier_ref_pts - cv2.perspectiveTransform(outlier_pts, M)) ** 2)
rmse_out = np.sqrt(mse_out)
print('RMSE (outliers):', rmse_out)
# 显示配准后的影像
aligned_img = cv2.warpPerspective(tgt_img, M, (ref_img.shape[1], ref_img.shape[0]))
plt.subplot(1, 2, 1)
plt.imshow(ref_img, cmap='gray')
plt.title('Reference Image')
plt.subplot(1, 2, 2)
plt.imshow(aligned_img, cmap='gray')
plt.title('Aligned Image')
plt.show()
```
在代码中,我们使用cv2.findHomography函数进行多项式拟合,并传入cv2.RANSAC作为参数,启用RANSAC算法。函数返回一个变换矩阵和一个掩膜,掩膜标记了哪些特征点被用于拟合,哪些特征点被认为是外部点。我们根据掩膜筛选出内部特征点和外部特征点,并计算内符合精度和外符合精度。最后,代码显示了配准前后的影像。
需要注意的是,在使用RANSAC算法时,需要选择合适的阈值。阈值越小,选择的内部特征点越少,精度越高,但可能会漏选一些内部点;阈值越大,选择的内部特征点越多,精度越低,但可能会将一些外部点误判为内部点。
阅读全文
相关推荐


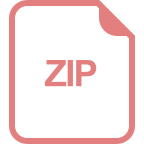
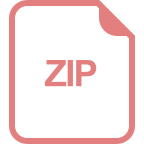
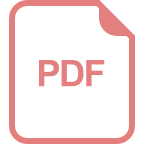
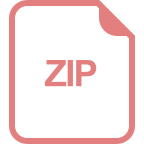
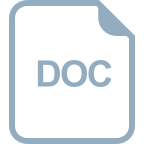
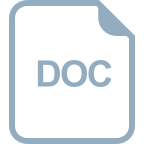
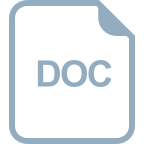
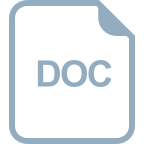
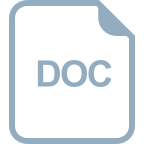
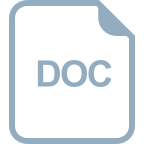
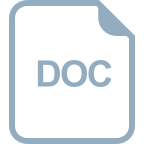
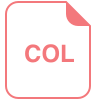
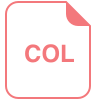

