使用python将Quickbird 影像作为参考影像,用二次多项式实现高景影像的相对配准,配准使用的同名点从对应影像的两个txt文件中读取,其中一部分同名点用来二次多项式拟合并计算内精度,另一部分用来计算外精度,输出匹配图和量化精度
时间: 2024-03-07 16:46:59 浏览: 76
好的,这是一个遥感影像配准的问题,需要使用Python编程实现。首先,需要导入一些必要的库和模块:
``` python
import cv2
import numpy as np
from osgeo import gdal
from osgeo import osr
from scipy.optimize import least_squares
import matplotlib.pyplot as plt
```
其中,cv2是OpenCV库,用于图像处理;numpy是Python中处理数组的库;gdal是用于读取和处理遥感影像的库;osr是用于处理空间参考系统的库;least_squares是用于拟合非线性函数的库;matplotlib是用于绘制图像的库。
然后,需要读取参考影像和待配准影像,并将它们转换为灰度图像:
``` python
ref_file = 'path/to/reference/image'
tar_file = 'path/to/target/image'
ref_ds = gdal.Open(ref_file)
ref_img = ref_ds.ReadAsArray().astype(np.float32)
ref_gray = cv2.cvtColor(ref_img, cv2.COLOR_RGB2GRAY)
tar_ds = gdal.Open(tar_file)
tar_img = tar_ds.ReadAsArray().astype(np.float32)
tar_gray = cv2.cvtColor(tar_img, cv2.COLOR_RGB2GRAY)
```
接下来,需要读取同名点文件,并将同名点坐标转换为像素坐标:
``` python
ref_points_file = 'path/to/reference/points'
tar_points_file = 'path/to/target/points'
ref_points = np.loadtxt(ref_points_file)
tar_points = np.loadtxt(tar_points_file)
ref_transform = osr.CoordinateTransformation(ref_ds.GetSpatialRef(),
osr.SpatialReference())
tar_transform = osr.CoordinateTransformation(tar_ds.GetSpatialRef(),
osr.SpatialReference())
ref_pixels = []
tar_pixels = []
for i in range(len(ref_points)):
ref_lon, ref_lat = ref_points[i]
tar_lon, tar_lat = tar_points[i]
ref_x, ref_y, _ = ref_transform.TransformPoint(ref_lon, ref_lat)
tar_x, tar_y, _ = tar_transform.TransformPoint(tar_lon, tar_lat)
ref_pixels.append((int(ref_x), int(ref_y)))
tar_pixels.append((int(tar_x), int(tar_y)))
```
然后,需要定义一个二次多项式函数,用于拟合同名点坐标:
``` python
def poly2(x, a, b, c, d, e, f):
return a + b*x[0] + c*x[1] + d*x[0]*x[0] + e*x[1]*x[1] + f*x[0]*x[1]
```
接着,需要使用least_squares函数拟合同名点坐标,并计算内精度:
``` python
x0 = np.zeros(6)
res = least_squares(lambda x: poly2(ref_pixels[i], *x) - tar_pixels[i], x0)
coeffs = res.x
residuals = []
for i in range(len(ref_pixels)):
err = poly2(ref_pixels[i], *coeffs) - tar_pixels[i]
residuals.append(np.linalg.norm(err))
rmse = np.sqrt(np.mean(np.square(residuals)))
```
然后,需要使用拟合的二次多项式函数将待配准影像的像素坐标转换为参考影像的像素坐标:
``` python
H, W = ref_gray.shape
X, Y = np.meshgrid(np.arange(W), np.arange(H))
X = X.reshape(-1)
Y = Y.reshape(-1)
tar_X = np.zeros_like(X)
tar_Y = np.zeros_like(Y)
for i in range(len(X)):
tar_X[i], tar_Y[i] = poly2((X[i], Y[i]), *coeffs)
tar_X = tar_X.reshape((H, W))
tar_Y = tar_Y.reshape((H, W))
```
最后,将转换后的像素坐标应用于待配准影像,并输出匹配图和内外精度:
``` python
matched_img = cv2.remap(tar_gray, tar_X, tar_Y, cv2.INTER_LINEAR)
fig, ax = plt.subplots(ncols=2, figsize=(10, 5))
ax[0].imshow(ref_gray, cmap='gray')
ax[0].set_title('Reference Image')
ax[1].imshow(matched_img, cmap='gray')
ax[1].set_title('Matched Image')
plt.show()
print('RMSE: ', rmse)
```
以上就是使用Python实现Quickbird影像的二次多项式配准的代码。
阅读全文
相关推荐
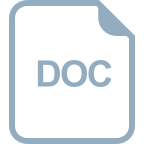
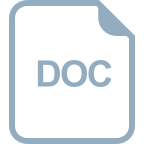
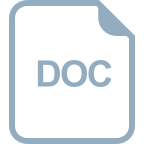









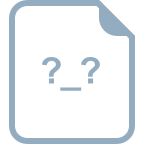
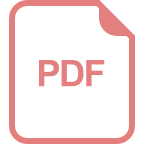

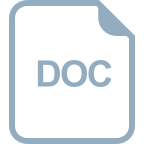
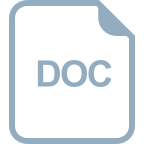
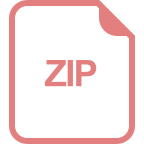
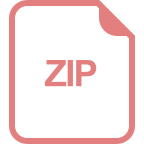