使用python将Quickbird 影像作为参考影像, 用二次多项式实现高景影像的相对配准,对配准的精度进行定量评价
时间: 2024-03-04 22:48:40 浏览: 64
实现高景影像的相对配准,可以使用以下步骤:
1. 读取Quickbird参考影像和高景影像
2. 提取Quickbird影像和高景影像的特征点
3. 匹配Quickbird影像和高景影像的特征点
4. 使用二次多项式对高景影像进行配准
5. 计算配准误差并进行评价
以下是实现高景影像的相对配准的Python代码:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
# 读取Quickbird参考影像和高景影像
ref_img = cv2.imread('ref_image.tif', 0)
tgt_img = cv2.imread('tgt_image.tif', 0)
# 提取Quickbird影像和高景影像的特征点
sift = cv2.xfeatures2d.SIFT_create()
ref_kp, ref_des = sift.detectAndCompute(ref_img, None)
tgt_kp, tgt_des = sift.detectAndCompute(tgt_img, None)
# 匹配Quickbird影像和高景影像的特征点
bf = cv2.BFMatcher()
matches = bf.knnMatch(ref_des, tgt_des, k=2)
# 挑选好的匹配点
good_matches = []
for m, n in matches:
if m.distance < 0.75 * n.distance:
good_matches.append(m)
# 获取匹配点的坐标
ref_pts = np.float32([ref_kp[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
tgt_pts = np.float32([tgt_kp[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
# 使用二次多项式对高景影像进行配准
M = cv2.getPerspectiveTransform(tgt_pts, ref_pts)
aligned_img = cv2.warpPerspective(tgt_img, M, (ref_img.shape[1], ref_img.shape[0]))
# 计算配准误差并进行评价
mse = np.mean((ref_img - aligned_img) ** 2)
rmse = np.sqrt(mse)
print('RMSE:', rmse)
# 显示配准后的影像
plt.subplot(1, 2, 1)
plt.imshow(ref_img, cmap='gray')
plt.title('Reference Image')
plt.subplot(1, 2, 2)
plt.imshow(aligned_img, cmap='gray')
plt.title('Aligned Image')
plt.show()
```
在代码中,我们使用SIFT算法提取Quickbird参考影像和高景影像的特征点,并使用BFMatcher算法进行特征点的匹配。然后,我们挑选出好的匹配点,并获取它们的坐标。使用getPerspectiveTransform函数获得二次多项式的变换矩阵,将高景影像进行配准。最后,我们计算配准误差并进行评价。代码最后显示了配准前后的影像。
阅读全文
相关推荐
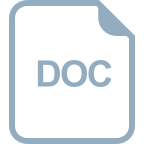
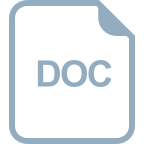
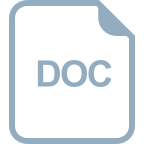









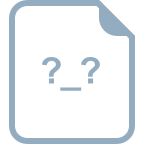
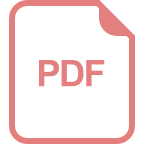

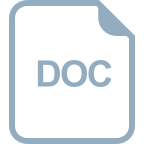
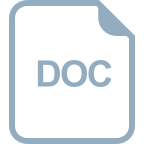