图书管理系统实现借书,还书以及数据的统计C语言
时间: 2024-03-01 13:53:57 浏览: 19
好的,这是一个相对比较复杂的问题,需要涉及到数据结构和文件操作。不过我会尽力回答你的问题。
首先,我们需要定义图书的数据结构,可以使用结构体来实现:
```c
typedef struct {
char name[50];
char author[50];
int num;
int borrowed_num;
} Book;
```
其中,`name`表示书名,`author`表示作者,`num`表示该书总共的数量,`borrowed_num`表示目前被借出去的数量。
接下来,我们需要将图书信息存储在文件中,可以使用文件操作来实现。我们可以将图书信息存储在一个文本文件中,每一行表示一本书,每一行的信息用逗号隔开,例如:
```
计算机网络,谢希仁,10,2
C程序设计语言, Brian W.Kernighan,15,5
```
其中,第一列为书名,第二列为作者,第三列为总共的数量,第四列为目前被借出去的数量。
接下来,我们来实现图书的借书和还书功能。具体代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100 // 最多支持100本书
typedef struct {
char name[50];
char author[50];
int num;
int borrowed_num;
} Book;
// 读取图书信息文件
int read_books(Book *books) {
FILE *fp = fopen("books.txt", "r");
if (fp == NULL) {
printf("Failed to read books file.\n");
return -1;
}
int i = 0;
char line[100];
while (fgets(line, sizeof(line), fp) != NULL) {
char *p = strtok(line, ",");
strcpy(books[i].name, p);
p = strtok(NULL, ",");
strcpy(books[i].author, p);
p = strtok(NULL, ",");
books[i].num = atoi(p);
p = strtok(NULL, ",");
books[i].borrowed_num = atoi(p);
i++;
}
fclose(fp);
return i;
}
// 写入图书信息文件
void write_books(Book *books, int num_books) {
FILE *fp = fopen("books.txt", "w");
if (fp == NULL) {
printf("Failed to write books file.\n");
return;
}
for (int i = 0; i < num_books; i++) {
fprintf(fp, "%s,%s,%d,%d\n", books[i].name, books[i].author, books[i].num, books[i].borrowed_num);
}
fclose(fp);
}
// 查找图书
int find_book(Book *books, int num_books, const char *name) {
for (int i = 0; i < num_books; i++) {
if (strcmp(books[i].name, name) == 0) {
return i;
}
}
return -1;
}
// 借书
void borrow_book(Book *books, int num_books, const char *name) {
int index = find_book(books, num_books, name);
if (index == -1) {
printf("Cannot find book %s.\n", name);
return;
}
if (books[index].num <= books[index].borrowed_num) {
printf("No available books of %s.\n", name);
return;
}
books[index].borrowed_num++;
printf("Borrow book %s successfully.\n", name);
write_books(books, num_books);
}
// 还书
void return_book(Book *books, int num_books, const char *name) {
int index = find_book(books, num_books, name);
if (index == -1) {
printf("Cannot find book %s.\n", name);
return;
}
if (books[index].borrowed_num == 0) {
printf("No borrowed books of %s.\n", name);
return;
}
books[index].borrowed_num--;
printf("Return book %s successfully.\n", name);
write_books(books, num_books);
}
// 统计图书信息
void summary_books(Book *books, int num_books) {
int total_num = 0;
int total_borrowed_num = 0;
for (int i = 0; i < num_books; i++) {
total_num += books[i].num;
total_borrowed_num += books[i].borrowed_num;
}
printf("Total number of books: %d\n", total_num);
printf("Total number of borrowed books: %d\n", total_borrowed_num);
}
int main() {
Book books[MAX_BOOKS];
int num_books = read_books(books);
// 借书示例
borrow_book(books, num_books, "计算机网络");
// 还书示例
return_book(books, num_books, "计算机网络");
// 统计图书信息
summary_books(books, num_books);
return 0;
}
```
在上面的代码中,`read_books()`函数用于读取图书信息文件,`write_books()`函数用于写入图书信息文件。`find_book()`函数用于查找图书,`borrow_book()`函数用于借书,`return_book()`函数用于还书,`summary_books()`函数用于统计图书信息。在`main()`函数中,我们演示了如何借书、还书和统计图书信息。
希望对你有所帮助!
相关推荐
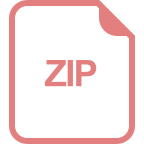
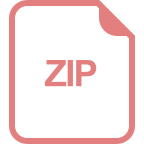
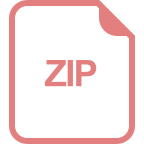













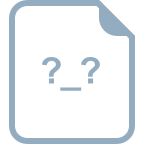