C++给出年份,打印该年年历,三个月为一行,要求一行一行打印
时间: 2023-08-17 19:05:05 浏览: 86
以下是一个简单的C++程序,可以根据输入的年份打印该年份的日历:
```
#include <iostream>
using namespace std;
// 判断是否为闰年
bool isLeapYear(int year) {
return ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0);
}
// 打印日历
void printCalendar(int year) {
int daysInMonth[] = {31, 28 + isLeapYear(year), 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; // 每个月的天数
string monthNames[] = {"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"}; // 月份名称
// 打印日历头部
cout << " " << year << endl;
cout << "---------------------------" << endl;
cout << " Jan Feb Mar Apr May Jun" << endl;
cout << "---------------------------" << endl;
// 打印每一行的三个月
for (int month = 0; month < 12; month += 3) {
for (int day = 1; day <= daysInMonth[month]; day++) {
// 打印日期
printf("%4d", day);
// 打印第二个月的日期
if (day == 1 && month == 0) {
for (int i = 1; i <= 9; i++) {
printf(" ");
}
for (int i = 1; i <= daysInMonth[month + 1]; i++) {
printf("%4d", i);
}
}
// 打印第三个月的日期
if (day == 1 && month == 0 || day == 1 && month == 3 || day == 1 && month == 6 || day == 1 && month == 9) {
for (int i = 1; i <= 9; i++) {
printf(" ");
}
for (int i = 1; i <= daysInMonth[month + 2]; i++) {
printf("%4d", i);
}
}
// 换行
if ((day + (month % 3 == 2 ? 31 : 0)) % 7 == 0) {
cout << endl;
}
}
cout << endl;
}
}
int main() {
int year;
cout << "请输入年份:";
cin >> year;
printCalendar(year);
return 0;
}
```
该程序使用了 isLeapYear 函数来判断是否为闰年,使用了两个数组来存储每个月的天数和月份名称。在打印日历时,首先打印日历头部,然后使用两个嵌套的 for 循环来遍历每个月的天数,并且根据需要打印第二个和第三个月的日期。最后,使用一个判断语句来换行,以实现一行一行打印的效果。
阅读全文
相关推荐
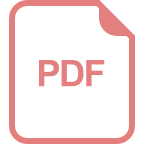
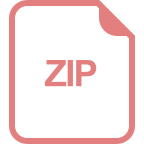
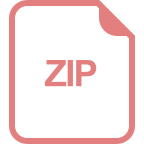
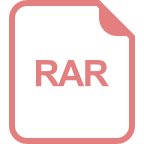
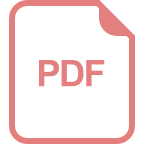
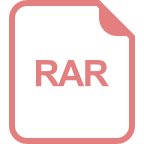
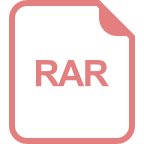
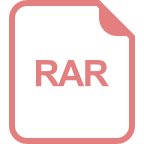
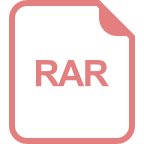
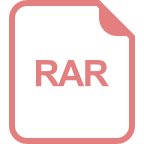
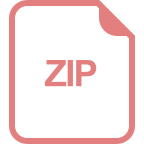
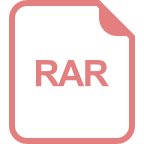
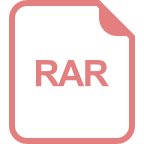
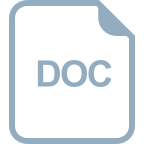