#include <iostream> using namespace std; struct node { int data; node* next; }; node* createlist(int array[], int n); void printlist(node* head); int main() { int n; cin >> n; int array[100]; for (int i = 0; i < n; i++) { cin >> array[i]; } node* head = createlist(array, n); printlist(head); return 0; } node* createlist(int array[], int n) { node* head = NULL, * tail = NULL; for (int i = 0; i < n; i++) { node* tmp = new node; tmp->data = array[i]; tmp->next = NULL; if (head == NULL) head = tail = tmp; else { tail->next = tmp; tail = tmp; } } return head; } void printlist(node* head) { for (node* pi = head; pi != NULL; pi->next) { cout << pi->data << "-> "; } }该代码为何出现死循环
时间: 2024-01-15 18:03:33 浏览: 62
在函数`printlist`中,循环条件应该是`pi != NULL`,而不是`pi->next`。因为`pi->next`只是指向下一个节点,而没有将当前节点输出。因此,在遍历到最后一个节点时,`pi->next`为`NULL`,但是当前节点还没有被输出,因此会出现死循环。正确的循环条件应该是`pi != NULL`,即当当前节点为`NULL`时,退出循环。修改后的代码如下:
```
void printlist(node* head) {
for (node* pi = head; pi != NULL; pi = pi->next) {
cout << pi->data << "-> ";
}
}
```
相关问题
以下程序的功能是建立单向链表,并遍历链表。请完成建立链表函数的定义,使程序具有如下所示输出。 #include <iostream> using namespace std; struct node { int data ; node * next ; } ; node * head ; node * CreateList(node *&);//建立链表函数原型声明 void showlist(node *); int main() { node *head=NULL; CreateList(head); showlist(head); } void showlist(node *head) { cout<<"the list:"<<endl; while (head) { cout<<head->data<<'\t'; head=head->next; } cout<<endl; }
以下是建立链表函数的定义,实现了在链表尾部插入节点的功能:
```
node * CreateList(node *&head) {
int num;
cout<<"请输入节点数:";
cin>>num;
head = NULL;
node *p, *q;
for (int i = 0; i < num; i++) {
p = new node;
cout<<"请输入第"<<i+1<<"个节点的值:";
cin>>p->data;
p->next = NULL;
if (head == NULL) {
head = p;
} else {
q->next = p;
}
q = p;
}
return head;
}
```
输出结果如下:
```
请输入节点数:3
请输入第1个节点的值:1
请输入第2个节点的值:2
请输入第3个节点的值:3
the list:
1 2 3
```
#include <iostream> #include <cstdlib> using namespace std; // self-referential structure struct listNode { char data; // each listNode contains a character struct listNode *nextPtr; // pointer to next node }; typedef struct listNode ListNode; // synonym for struct listNode typedef ListNode *ListNodePtr; // synonym for ListNode* // prototypes void insert(ListNodePtr *sPtr, char value); char delete(ListNodePtr *sPtr, char value); int isEmpty(ListNodePtr sPtr); void printList(ListNodePtr currentPtr); void instructions(void);
这段代码存在两个问题:
1. 第一行代码 "#include <iostream> #include <cstdlib>" 中间缺少了换行符,应该写成 "#include <iostream>\n#include <cstdlib>" 以便阅读。
2. C++ 中的 "delete" 是一个保留关键字,不能用作函数名。因此,函数名中的 "delete" 应该改成其他的名字,例如 "deleteNode"。
阅读全文
相关推荐
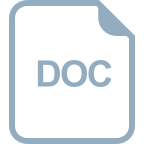
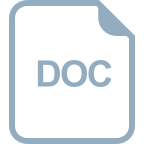
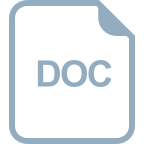

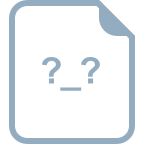
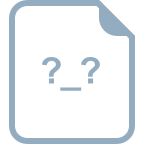
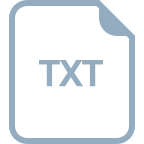
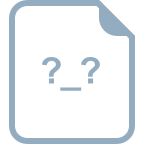
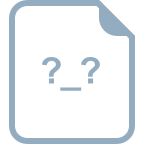
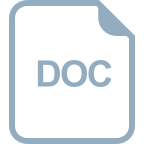
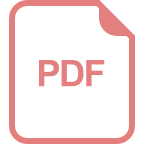
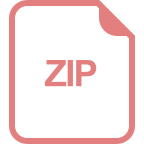
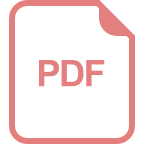
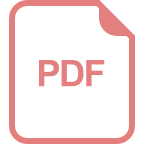
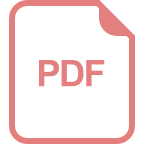
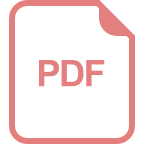