如何将卷积神经网络的分类层的输出以数据格式输出
时间: 2024-05-16 08:14:38 浏览: 13
要将卷积神经网络的分类层的输出以数据格式输出,可以使用以下步骤:
1. 在代码中定义一个测试模型(test model),该模型应该与训练模型(train model)的结构相同,只是去掉了训练时使用的 Dropout 层等一些层。
2. 加载训练好的模型权重文件。
3. 准备测试集数据并进行预处理,例如归一化、调整尺寸等。
4. 使用测试模型对测试集数据进行预测,得到分类层的输出。
5. 将分类层的输出转化为数据格式输出,例如使用 numpy 库进行转化。
下面是一个示例代码,它假设你已经定义了一个名为 test_model 的测试模型,并加载了训练好的模型权重文件。这个示例代码使用 CIFAR-10 数据集进行测试,并将分类层的输出以 numpy 数组的形式输出。
```python
import numpy as np
from keras.datasets import cifar10
from keras.utils import np_utils
from keras.models import load_model
# 加载 CIFAR-10 数据集
(X_train, y_train), (X_test, y_test) = cifar10.load_data()
# 预处理数据
X_test = X_test.astype('float32')
X_test /= 255
y_test = np_utils.to_categorical(y_test, 10)
# 加载训练好的模型权重
test_model = load_model('my_model.h5')
# 对测试集进行预测
y_pred = test_model.predict(X_test)
# 将分类层的输出转化为数据格式输出
y_pred_classes = np.argmax(y_pred, axis=1)
# 输出测试集的准确率
accuracy = np.mean(y_pred_classes == y_test)
print('Accuracy:', accuracy)
```
在上面的代码中,我们使用了 `np.argmax()` 函数来获取每个样本的预测标签,即分类层的输出。然后,我们使用 `np.mean()` 函数来计算测试集的准确率。
相关推荐
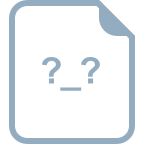
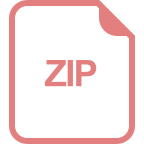
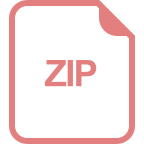














