怎么用python将一个csv文件数据绘制成折线图
时间: 2024-05-08 19:19:19 浏览: 13
可以使用Python中的Matplotlib库来绘制折线图。以下是一个简单的示例代码:
```python
import matplotlib.pyplot as plt
import pandas as pd
# 读取CSV文件
data = pd.read_csv('file.csv')
# 提取数据列
x_values = data['x_column']
y_values = data['y_column']
# 绘制折线图
plt.plot(x_values, y_values)
# 添加标题和标签
plt.title('Title of the Plot')
plt.xlabel('X Axis Label')
plt.ylabel('Y Axis Label')
# 显示图像
plt.show()
```
请将“file.csv”替换为您的CSV文件路径,并将“x_column”和“y_column”替换为您的数据列名称。
相关问题
Python如何将csv文件绘制为折线图
使用Python中的Matplotlib库可以轻松地将CSV文件绘制成折线图。以下是实现此目标的一些步骤:
1. 导入必要的库
```python
import pandas as pd
import matplotlib.pyplot as plt
```
2. 使用Pandas库读取CSV文件,并将其存储在DataFrame中
```python
df = pd.read_csv('file.csv')
```
3. 在DataFrame中选择要绘制的列
```python
x = df['column_x']
y = df['column_y']
```
4. 使用Matplotlib绘制折线图
```python
plt.plot(x, y)
plt.title('Title of the Plot')
plt.xlabel('X Label')
plt.ylabel('Y Label')
plt.show()
```
这样就可以将CSV文件绘制为折线图了。你可以根据自己的需求修改标题、坐标轴标签等属性。
用python将csv文件中的数据化成折线图
下面是一个简单的示例代码,使用pandas和matplotlib库将csv文件中的数据可视化成折线图:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 读取csv文件
data = pd.read_csv('data.csv')
# 设置x轴和y轴数据
x = data['x']
y = data['y']
# 绘制折线图
plt.plot(x, y)
# 设置图表标题和x轴、y轴标签
plt.title('Line chart')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
# 显示图表
plt.show()
```
其中,data.csv是一个包含两列数据的csv文件,第一列是x轴数据,第二列是y轴数据。运行该代码,将生成一个折线图,横轴为x轴数据,纵轴为y轴数据。可以根据需要对图表进行进一步的设置和美化。
相关推荐
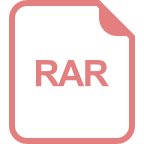
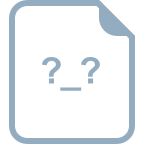
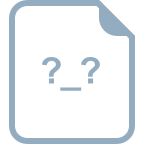












