C语言编写程序读取网页文件(例如:a.htm),找出标题内容以及在文件中行数写入文件result.txt中,同时统计标题中数字字符和字母字符的个数(分别编写函数实现统计),也写入文件result.txt中,result.txt中写入的格式如下: a.htm网页的标题是: 标题在文件 a.htm网页的第几行: 标题中数字字符的个数: 标题中字母字符的个数: 注意:网页文件(例如:a.htm)每行最多255个字符。 要求: (1)输入输出要有文字说明,例如:读文件xxx等; (2)程序代码的重要部分要有注释; (3)代码结构清晰,有缩进格式; (4)有程序调试过
时间: 2023-12-03 15:42:06 浏览: 80
以下是C语言编写程序读取网页文件并统计标题内容的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAXLINE 255 // 每行最多255个字符
// 统计字符串中数字字符的个数
int count_digits(char *str) {
int count = 0;
while (*str) {
if (isdigit(*str)) {
count++;
}
str++;
}
return count;
}
// 统计字符串中字母字符的个数
int count_letters(char *str) {
int count = 0;
while (*str) {
if (isalpha(*str)) {
count++;
}
str++;
}
return count;
}
int main() {
char filename[100];
printf("请输入文件名:");
scanf("%s", filename);
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("文件打开失败!\n");
exit(1);
}
char line[MAXLINE]; // 存储每行的内容
char title[MAXLINE]; // 存储标题内容
int line_num = 0; // 存储标题所在的行数
int digits_count = 0; // 标题中数字字符的个数
int letters_count = 0; // 标题中字母字符的个数
while (fgets(line, MAXLINE, fp) != NULL) {
line_num++;
// 查找标题
char *pos = strstr(line, "<title>");
if (pos != NULL) {
pos += strlen("<title>"); // 跳过<title>标签
char *endpos = strstr(pos, "</title>");
if (endpos != NULL) {
*endpos = '\0'; // 截断字符串
strcpy(title, pos); // 复制标题内容
}
// 统计标题中数字字符和字母字符的个数
digits_count = count_digits(title);
letters_count = count_letters(title);
break; // 找到标题后结束循环
}
}
fclose(fp);
// 将结果输出到文件
FILE *outfp = fopen("result.txt", "w");
if (outfp == NULL) {
printf("输出文件打开失败!\n");
exit(1);
}
fprintf(outfp, "%s网页的标题是:%s\n", filename, title);
fprintf(outfp, "标题在文件 %s 网页的第%d行\n", filename, line_num);
fprintf(outfp, "标题中数字字符的个数:%d\n", digits_count);
fprintf(outfp, "标题中字母字符的个数:%d\n", letters_count);
fclose(outfp);
printf("结果已输出到文件result.txt中!\n");
return 0;
}
```
程序的逻辑比较简单,首先让用户输入要读取的文件名,然后打开文件并逐行读取文件内容。在读取的过程中查找标题内容,找到后记录标题所在的行数以及标题中数字字符和字母字符的个数。最后将结果输出到文件result.txt中。
相关推荐












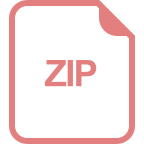
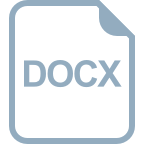
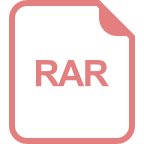