用C语言写出一个高性能线程
时间: 2024-05-14 14:12:32 浏览: 108
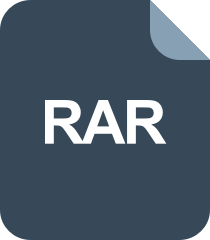
C语言线程编程

因为线程的高性能与多线程编程的复杂性关系密切,因此我们需要考虑一些关键因素来编写高性能的线程,例如线程的创建,上下文切换,锁的使用,以及线程的同步和通信等等。
以下是一个使用C语言编写的高性能线程示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 10
void* thread_func(void* arg) {
int tid = *(int*)arg;
printf("Hello from thread %d\n", tid);
pthread_exit(NULL);
}
int main() {
pthread_t threads[NUM_THREADS];
int thread_args[NUM_THREADS];
for (int i = 0; i < NUM_THREADS; i++) {
thread_args[i] = i;
int rc = pthread_create(&threads[i], NULL, thread_func, &thread_args[i]);
if (rc) {
printf("ERROR: return code from pthread_create() is %d\n", rc);
exit(1);
}
}
for (int i = 0; i < NUM_THREADS; i++) {
pthread_join(threads[i], NULL);
}
printf("All threads completed. Exiting.\n");
pthread_exit(NULL);
}
```
上面的代码创建了10个线程,每个线程都会打印出自己的线程ID,并且在完成后退出。
在代码中,我们使用了pthread库来创建和管理线程。在main函数中,我们首先声明了10个线程(pthread_t类型),以及10个整型数组来存储每个线程的参数。然后,我们使用for循环来创建每个线程,并将其参数设置为对应的整数i。在创建线程时,我们使用了pthread_create函数,该函数接受四个参数:一个指向线程标识符的指针,线程的属性(通常为NULL),指向线程函数的指针,以及指向线程参数的指针。在这个示例中,我们将线程函数设置为thread_func,线程参数设置为thread_args[i]的地址。
在thread_func函数中,我们首先获取线程ID(tid),然后打印出“Hello from thread”和tid。最后,我们使用pthread_exit函数来退出线程。
在main函数中,我们使用pthread_join函数来等待所有线程完成。这确保了所有线程都已经退出,然后我们打印出“All threads completed. Exiting.”。最后,我们使用pthread_exit函数来退出程序。
这个线程示例使用了最基本的线程创建和管理技术。要编写更高性能的线程,我们需要考虑更多的因素,例如线程池,线程同步和通信,以及避免竞争条件等等。
阅读全文
相关推荐
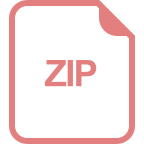
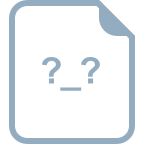
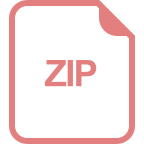
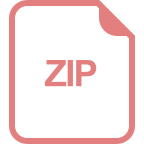
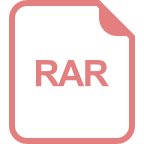
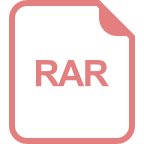
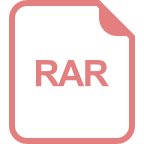
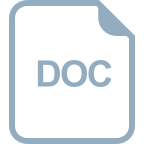
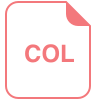
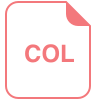
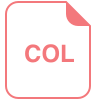
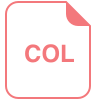
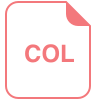
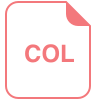
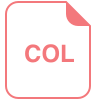
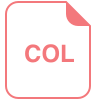
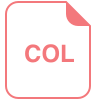