多线程编程基础:掌握C语言中的线程库
发布时间: 2024-01-18 08:20:24 阅读量: 50 订阅数: 45 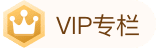
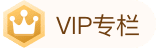
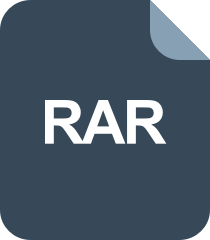
基于C语言实现的C语言线程库(含源码)

# 1. 第一章 引言
## 1.1 什么是多线程编程
多线程编程是指在一个程序中同时运行多个线程,每个线程有自己的独立执行路径。多线程编程可以充分利用多核处理器的并行处理能力,提高程序的执行效率。
## 1.2 为什么需要多线程编程
随着计算机硬件的快速发展,单个处理器的速度已经不能满足日益复杂的应用需求。多线程编程可以将任务分解成多个更小的子任务,由多个线程并行执行,提高程序的响应速度和处理能力。
## 1.3 C语言中的线程库介绍
在C语言中,可以使用POSIX线程库(Pthreads)来进行多线程编程。Pthreads提供了对多线程的支持,包括线程的创建、销毁、同步与互斥、线程通信与数据共享等功能。
Pthreads库提供了一组函数和数据类型,可以方便地操作线程相关的操作。在使用Pthreads库时,需要包含头文件`<pthread.h>`,并链接相应的线程库。
接下来,我们将详细介绍多线程编程的各个方面内容。
# 2. 线程的创建与销毁
在多线程编程中,线程的创建和销毁是非常重要的操作。本章将详细介绍如何创建和销毁线程,并展示如何创建多个线程。
### 2.1 线程的创建
在C语言中,我们可以使用`pthread_create`函数来创建一个新的线程。该函数的原型如下:
```c
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine)(void*), void *arg);
```
- `thread`:用于存储新线程ID的指针。
- `attr`:线程的属性(可选参数),一般设置为`NULL`。
- `start_routine`:线程的入口函数,即线程要执行的任务。
- `arg`:传递给线程入口函数的参数。
下面是一个简单的例子,演示如何创建一个线程:
```c
#include <stdio.h>
#include <pthread.h>
void* thread_func(void* arg) {
int i;
for (i = 0; i < 5; i++) {
printf("Thread: %d\n", i);
sleep(1);
}
pthread_exit(NULL);
}
int main() {
pthread_t thread;
int ret = pthread_create(&thread, NULL, thread_func, NULL);
if (ret) {
printf("Error: pthread_create() failed\n");
return 1;
}
pthread_join(thread, NULL);
return 0;
}
```
在上述示例中,我们定义了一个线程入口函数`thread_func`,该函数会打印出线程的编号,并在每次打印后等待1秒钟。在`main`函数中,我们使用`pthread_create`创建了一个线程,并传入`thread_func`作为线程的入口函数。`pthread_join`函数用于等待线程的结束。
### 2.2 线程的销毁
在线程的工作完成后,我们需要对线程进行销毁,释放相关的资源。在C语言中,我们可以使用`pthread_exit`函数或`pthread_cancel`函数来实现线程的销毁。
- `pthread_exit`:用于线程主动退出,其原型如下:
```c
void pthread_exit(void *retval);
```
- `pthread_cancel`:用于请求取消一个线程,即将线程标记为“取消请求”状态,由线程自行决定在何时取消。其原型如下:
```c
int pthread_cancel(pthread_t thread);
```
下面是一个简单的例子,演示如何使用`pthread_exit`函数来销毁线程:
```c
#include <stdio.h>
#include <pthread.h>
void* thread_func(void* arg) {
int i;
for (i = 0; i < 5; i++) {
printf("Thread: %d\n", i);
sleep(1);
}
pthread_exit(NULL);
}
int main() {
pthread_t thread;
int ret = pthread_create(&thread, NULL, thread_func, NULL);
if (ret) {
printf("Error: pthread_create() failed\n");
return 1;
}
pthread_join(thread, NULL);
printf("Main Thread Exiting\n");
return 0;
}
```
在上述示例中,线程在完成5次打印后调用了`pthread_exit(NULL)`来退出线程,并在`main`函数中用`pthread_join`等待线程的结束。最后,主线程打印了一条消息表示自己的退出。
### 2.3 创建多个线程
除了创建单个线程,在多线程编程中,创建多个线程也是非常常见的操作。我们可以使用循环结构来创建多个线程,每个线程执行不同的任务。
下面是一个简单的例子,演示如何创建多个线程:
```c
#include <stdio.h>
#include <pthread.h>
#define NUM_THREADS 5
void* thread_func(void* arg) {
int tid = *((int*) arg);
printf("Thread ID: %d\n", tid);
pthread_exit(NULL);
}
int main() {
pthread_t threads[NUM_THREADS];
int thread_ids[NUM_THREADS];
int i;
for (i = 0; i < NUM_THREADS; i++) {
thread_ids[i] = i;
int ret = pthread_create(&threads[i], NULL, thread_func, (void*) &thread_ids[i]);
if (ret) {
printf("Error: pthread_create() failed\n");
return 1;
}
}
for (i = 0; i < NUM_THREADS; i++) {
pthread_join(threads[i], NULL);
}
printf("Main Thread Exiting\n");
return 0;
}
```
在上述示例中,我们定义了一个线程入口函数`thread_func`,该函数会打印出线程的ID。在`main`函数中,我们循环创建了5个线程,并将线程ID作为参数传递给线程入口函数。最后,使用`pthread_join`等待所有线程的结束。
通过上述示例,我们可以看到如何在C语言中创建和销毁线程,并实现多个线程的同时执行。在实际应用中,我们可以根据具体需求来创建和管理线程,以提高程序的并发性和性能。
# 3. 线程同步与互斥
在多线程编程中,线程之间的同步与互斥是非常重要的概念。当多个线程并发访问共享资源时,往往需要进行同步操作以避免数据竞争和不确定的行为。下面将介绍互斥锁、条件变量和信号量的概念与使用。
#### 3.1 互斥锁的概念与使用
互斥锁(Mutex)是一种常用的线程同步机制,用于保护临界区资源,确保在同一时刻只有一个线程访问共享资源。在C语言中,互斥锁的使用通常包括初始化、加锁和解锁操作。
```c
#include <stdio.h>
#include <pthread.h>
pthread_mutex_t mutex;
void* thread_function(void* arg) {
pthread_mutex_lock(&mutex); // 加锁
// 访问临界区资源
pthread_mutex_unlock(&mutex); // 解锁
return NULL;
}
int main() {
pthread_t tid;
pthread_mutex_init(&mutex, NULL); // 初始化互斥锁
pthread_create(&tid, NULL, thread_function, NULL);
// 其他操作
pthread_join(tid, NULL);
pthread_mutex_destroy(&mutex); // 销毁互斥锁
return 0;
}
```
在以上示例中,`pthread_mutex_lock`用于加锁,`pthread_mutex_unlock`用于解锁,确保同一时刻只有一个线程可以进入临界区。同时在主函数中,使用`pthread_mutex_init`进行互斥锁的初始化,`pthread_mutex_destroy`进行销毁。
#### 3.2 条件变量的概念与使用
条件变量(Condition Variable)通常与互斥锁配合使用,用于线程间的同步和通信,允许线程在某个条件成立时等待,并在条件发生变化时通知其他线程。在C语言中,条件变量的使用包括初始化、等待和通知操作。
```c
#include <stdio.h>
#include <pthread.
```
0
0
相关推荐
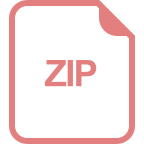
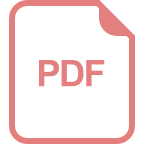
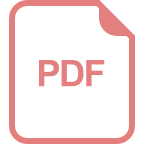
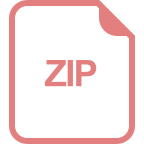
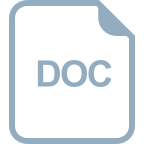
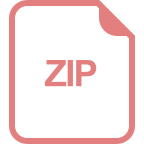
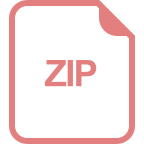