driverclassname怎么写
时间: 2023-09-15 12:05:30 浏览: 83
### 回答1:
driverclassname应该按照对应的数据库驱动程序来写,例如:
- MySQL数据库驱动程序的driverclassname为:com.mysql.jdbc.Driver
- Oracle数据库驱动程序的driverclassname为:oracle.jdbc.driver.OracleDriver
- SQL Server数据库驱动程序的driverclassname为:com.microsoft.sqlserver.jdbc.SQLServerDriver
需要根据具体的数据库驱动程序来确定driverclassname的写法。
### 回答2:
在Java中,如果想要指定数据库驱动程序的类名,可以使用`driverclassname`参数。`driverclassname`属性是用于加载数据库驱动程序的类名。根据不同的数据库,`driverclassname`的写法略有不同。
以MySQL为例,`driverclassname`属性应该设置为`com.mysql.jdbc.Driver`。这是因为MySQL官方提供的驱动程序类是`com.mysql.jdbc.Driver`。
对于Oracle数据库,`driverclassname`属性应该设置为`oracle.jdbc.driver.OracleDriver`。
对于SQL Server数据库,`driverclassname`属性应该设置为`com.microsoft.sqlserver.jdbc.SQLServerDriver`。
对于PostgreSQL数据库,`driverclassname`属性应该设置为`org.postgresql.Driver`。
其他数据库的驱动程序类名可以在它们的官方文档或驱动程序提供方的文档中找到。
需要注意的是,不同版本的数据库驱动程序对应的`driverclassname`可能会有所不同,因此建议在使用时仔细查阅相关文档确认正确的类名。
### 回答3:
在Java中,使用JDBC连接数据库时,需要通过设置driverclassname属性来指定要使用的数据库驱动程序类。driverclassname属性的值应该是数据库驱动程序类的全限定名。
例如,如果要连接MySQL数据库,则driverclassname属性的值应为"com.mysql.jdbc.Driver"。而如果要连接Oracle数据库,则driverclassname属性的值应为"oracle.jdbc.driver.OracleDriver"。
要正确设置driverclassname属性,需要先将相应的数据库驱动程序包导入到项目中。然后,在代码中通过Class.forName()方法加载相应的数据库驱动程序类。例如,如果要连接MySQL数据库,可以在代码中使用以下语句加载类:
Class.forName("com.mysql.jdbc.Driver");
通过以上操作,即可将driverclassname属性正确地设置为相应的数据库驱动程序类。
需要注意的是,在使用不同的数据库时,driverclassname属性的取值也不同。因此,在设置driverclassname时,需要根据所使用的数据库驱动程序类的全限定名来进行设置。这种设置方法可以确保正确加载并使用相应的数据库驱动程序。
综上所述,要正确设置driverclassname属性,应根据所使用的数据库驱动程序类的全限定名来进行设置,并在代码中加载相应的数据库驱动程序类。
相关推荐
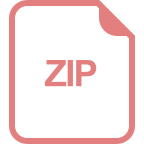
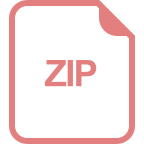
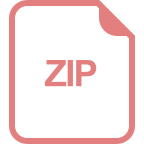














