numpy如何导入csv文件时如何处理第一行标题
时间: 2024-05-05 17:20:23 浏览: 109
可以使用numpy中的genfromtxt函数来导入csv文件。在函数的参数中设置delimiter为逗号,dtype为None,skip_header为1即可忽略第一行标题。代码示例如下:
```python
import numpy as np
data = np.genfromtxt('data.csv', delimiter=',', dtype=None, skip_header=1)
```
其中'data.csv'为需要导入的csv文件名。
相关问题
numpy读取csv文件
可以使用numpy中的`loadtxt`函数来读取csv文件。具体步骤如下:
1. 导入numpy库
```python
import numpy as np
```
2. 使用`loadtxt`函数读取csv文件
```python
data = np.loadtxt('filename.csv', delimiter=',', skiprows=1)
```
- `filename.csv`为csv文件路径和文件名。
- `delimiter=','`指定csv文件中的分隔符为逗号。
- `skiprows=1`表示跳过文件的第一行,通常第一行是标题行。
3. 数据处理
`loadtxt`函数返回一个numpy数组,可以对其进行进一步处理。
例如,如果csv文件包含了两列数据,可以通过以下方式将其分别存储到两个数组中:
```python
x = data[:, 0]
y = data[:, 1]
```
`:`表示选取整个数组,`0`和`1`表示选取第一列和第二列。
完整代码示例:
```python
import numpy as np
data = np.loadtxt('filename.csv', delimiter=',', skiprows=1)
x = data[:, 0]
y = data[:, 1]
print(x)
print(y)
```
numpy读取csv某一列
可以使用numpy的loadtxt函数来读取csv文件中的某一列数据。首先需要将csv文件加载为numpy数组,然后使用数组的索引来获取所需的列。
以下是一个读取csv文件某一列数据的示例代码:
```python
import numpy as np
# 加载csv文件为numpy数组
data = np.loadtxt('data.csv', delimiter=',', skiprows=1)
# 获取第二列数据
col2 = data[:, 1]
# 输出第二列数据
print(col2)
```
在上面的代码中,`loadtxt`函数用于加载csv文件。`delimiter`参数用于指定分隔符,`skiprows`参数用于跳过文件的第一行标题。然后使用`[:, 1]`来获取第二列数据,其中`:`表示选择所有行,`1`表示选择第二列。最后,输出所需的列数据即可。
相关推荐
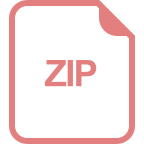
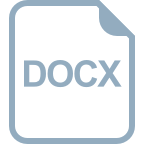
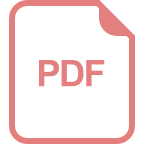












