Android实现多线程下载安装
时间: 2024-03-18 11:19:51 浏览: 105
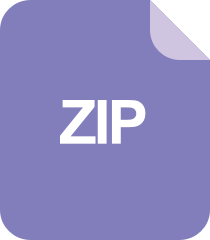
android 实现多线程下载。
Android 实现多线程下载安装主要需要以下步骤:
1. 获取下载链接和文件名:在下载之前,需要先获取下载链接和文件名。
2. 创建多个线程:每个线程负责下载文件的一部分。这里可以使用 Java 的线程池技术,通过线程池管理多个线程。
3. 下载文件:每个线程下载文件的一部分,并将下载的数据写入本地文件。
4. 合并文件:所有线程下载完成后,将下载的文件片段合并为完整的文件。
5. 安装应用:下载完成后,通过系统的 Intent 启动安装程序,将下载的应用安装到设备上。
下面是一个简单的示例代码:
```
public class DownloadTask {
private static final int THREAD_COUNT = 3; // 线程数量
private String downloadUrl; // 下载链接
private String fileName; // 文件名
private String savePath; // 保存路径
private long fileSize; // 文件大小
private Context context;
public DownloadTask(Context context, String downloadUrl, String fileName, String savePath) {
this.context = context;
this.downloadUrl = downloadUrl;
this.fileName = fileName;
this.savePath = savePath;
}
public void startDownload() {
try {
URL url = new URL(downloadUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setConnectTimeout(5000);
fileSize = connection.getContentLength();
connection.disconnect();
RandomAccessFile file = new RandomAccessFile(savePath + fileName, "rw");
file.setLength(fileSize);
file.close();
ExecutorService executor = Executors.newFixedThreadPool(THREAD_COUNT);
long blockSize = fileSize / THREAD_COUNT;
for (int i = 0; i < THREAD_COUNT; i++) {
long start = i * blockSize;
long end = (i + 1) * blockSize - 1;
if (i == THREAD_COUNT - 1) {
end = fileSize - 1;
}
DownloadThread thread = new DownloadThread(context, downloadUrl, savePath, fileName, start, end);
executor.execute(thread);
}
executor.shutdown();
while (!executor.isTerminated()) {
Thread.sleep(100);
}
mergeFile();
installApk();
} catch (Exception e) {
e.printStackTrace();
}
}
// 合并文件
private void mergeFile() throws IOException {
File file = new File(savePath + fileName);
FileOutputStream fos = new FileOutputStream(file);
for (int i = 0; i < THREAD_COUNT; i++) {
FileInputStream fis = new FileInputStream(savePath + fileName + ".part" + i);
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
fis.close();
new File(savePath + fileName + ".part" + i).delete();
}
fos.close();
}
// 安装应用
private void installApk() {
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setDataAndType(Uri.fromFile(new File(savePath + fileName)), "application/vnd.android.package-archive");
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
context.startActivity(intent);
}
}
class DownloadThread extends Thread {
private static final int BUFFER_SIZE = 1024;
private Context context;
private String downloadUrl;
private String savePath;
private String fileName;
private long start;
private long end;
public DownloadThread(Context context, String downloadUrl, String savePath, String fileName, long start, long end) {
this.context = context;
this.downloadUrl = downloadUrl;
this.savePath = savePath;
this.fileName = fileName;
this.start = start;
this.end = end;
}
@Override
public void run() {
try {
URL url = new URL(downloadUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setConnectTimeout(5000);
connection.setRequestProperty("Range", "bytes=" + start + "-" + end);
File file = new File(savePath + fileName + ".part" + getId());
FileOutputStream fos = new FileOutputStream(file);
InputStream is = connection.getInputStream();
byte[] buffer = new byte[BUFFER_SIZE];
int len;
while ((len = is.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
fos.close();
is.close();
connection.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在这个示例中,我们创建了一个 `DownloadTask` 类来管理下载任务。`startDownload()` 方法启动下载任务,首先获取文件大小,并创建一个与文件大小相同的空文件。然后使用线程池创建多个线程来下载文件的不同部分。每个线程下载完成后,将文件片段写入以该线程 ID 命名的临时文件中。所有线程结束后,将临时文件合并为完整的文件,并启动安装程序来安装应用。
阅读全文
相关推荐
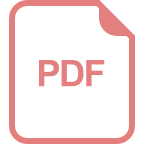
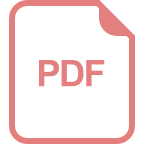
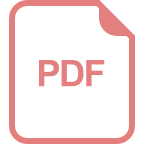
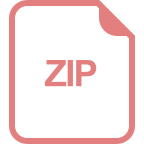
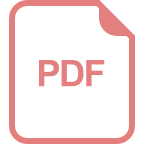
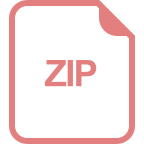
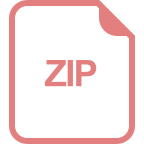
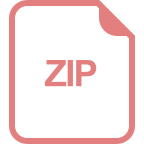
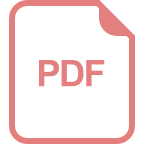
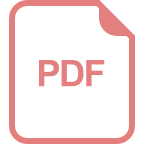
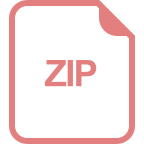
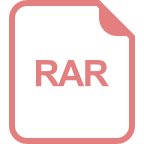
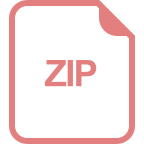
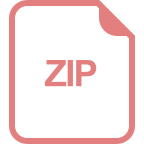
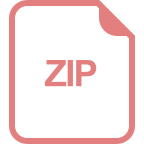