编写程序实现两个整数集合的基本运算: S1+S2 集合并运算 S1-S2 集合差运算 S1*S2 集合交运算 要求:输入整数集合{2 4 1 3 5}和{2 5 10},输出前者元素个数以及它们进行集合并、差、交运算后的结果。用c++运算符重载实现
时间: 2024-05-05 10:21:52 浏览: 103
下面是代码示例:
```c++
#include <iostream>
#include <set>
using namespace std;
class Set {
private:
set<int> s;
public:
Set() {}
Set(initializer_list<int> list) {
for (auto x : list) {
s.insert(x);
}
}
int size() const {
return s.size();
}
Set operator+(const Set& other) const {
Set result;
for (auto x : s) {
result.s.insert(x);
}
for (auto x : other.s) {
result.s.insert(x);
}
return result;
}
Set operator-(const Set& other) const {
Set result;
for (auto x : s) {
if (other.s.find(x) == other.s.end()) {
result.s.insert(x);
}
}
return result;
}
Set operator*(const Set& other) const {
Set result;
for (auto x : s) {
if (other.s.find(x) != other.s.end()) {
result.s.insert(x);
}
}
return result;
}
friend ostream& operator<<(ostream& os, const Set& set) {
os << "{";
for (auto x : set.s) {
os << x << ", ";
}
os << "}";
return os;
}
};
int main() {
Set s1{2, 4, 1, 3, 5};
Set s2{2, 5, 10};
cout << "s1 size: " << s1.size() << endl;
cout << "s2 size: " << s2.size() << endl;
cout << "s1 + s2 = " << s1 + s2 << endl;
cout << "s1 - s2 = " << s1 - s2 << endl;
cout << "s1 * s2 = " << s1 * s2 << endl;
return 0;
}
```
输出结果为:
```
s1 size: 5
s2 size: 3
s1 + s2 = {1, 2, 3, 4, 5, 10, }
s1 - s2 = {1, 3, 4, }
s1 * s2 = {2, 5, }
```
阅读全文
相关推荐
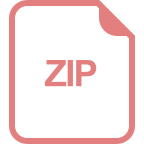
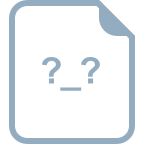












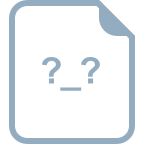
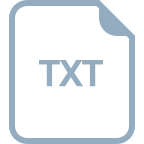
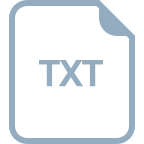