python中的np where_numpy中的np.where
时间: 2023-06-29 12:17:45 浏览: 110
`np.where` 是 NumPy 库中的一个函数,用于根据给定条件返回输入数组中满足条件的元素的索引或者值。它的使用方法如下:
```python
import numpy as np
a = np.array([1, 2, 3, 4, 5])
condition = a > 3
print(np.where(condition))
```
这段代码会输出 `[3, 4]`,表示 `a` 数组中大于 3 的元素的索引。`np.where` 函数的第一个参数是条件,第二个参数是满足条件时返回的值,第三个参数是不满足条件时返回的值。如果只有一个参数,那么函数会返回满足条件的元素的索引。
`where` 函数是 Python 内置的函数,也可以用于根据条件返回元素的索引或者值。但是,它的用法与 `np.where` 函数略有不同。例如:
```python
a = [1, 2, 3, 4, 5]
condition = [True, False, False, True, True]
print([i for i, x in enumerate(a) if condition[i]])
```
这段代码实现了与上面相同的功能,但是需要用列表推导式手动遍历列表并返回满足条件的元素的索引。相比之下,`np.where` 函数更加方便快捷。
相关问题
python中np.where
在Python中,np.where()函数用于返回满足条件的元素的下标。它可以接收一个参数,返回符合条件的下标,也可以接收三个参数,用于三目运算。\[1\]举个例子,如果我们有一个数组a = np.array(\[1, 2, 3, 1, 2, 3, 1, 2, 3\]),我们可以使用np.where(a > 2)来找到数组中大于2的元素的下标,结果为(array(\[2, 5, 8\], dtype=int32),)。\[1\]另外,我们也可以使用a\[a > 2\]来直接返回满足条件的元素,结果为array(\[3, 3, 3\])。\[1\]
此外,np.where()函数还可以接收两个参数,用于替换数组中的元素。\[3\]举个例子,如果我们有一个数组a = np.arange(12).reshape(\[3, 4\]),我们可以使用np.where(a > 5, 1, 0)来将数组中大于5的元素替换为1,不大于5的元素替换为0,结果为array(\[\[0, 0, 0, 0\], \[0, 0, 1, 1\], \[1, 1, 1, 1\]\])。\[3\]
#### 引用[.reference_title]
- *1* [python 中 np.where](https://blog.csdn.net/erinapple/article/details/80838359)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [Python numpy使用记录3.数组元素筛选,np.where](https://blog.csdn.net/qq_41035283/article/details/124092350)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
python中np.where函数
np.where函数是numpy库中的一个函数,它的作用是根据指定的条件,返回符合条件的元素的索引或值。np.where函数的语法如下:
np.where(condition[, x, y])
其中,condition是指定的条件,x和y是可选的参数,用于指定符合条件和不符合条件的返回值。
当只有condition参数时,np.where函数返回符合条件的元素的索引。
当有x和y参数时,np.where函数会根据condition的值,返回符合条件的元素的值或不符合条件的元素的值。
例如,以下代码会将数组a中小于0的元素替换为0,其他元素不变:
```python
import numpy as np
a = np.array([-1, 2, -3, 4, 5])
b = np.where(a < 0, 0, a)
print(b)
```
输出结果为:
```
[0 2 0 4 5]
```
其中,np.where(a < 0, 0, a)的作用是将数组a中小于0的元素替换为0,其他元素不变。
相关推荐
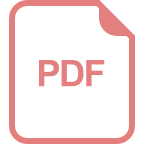
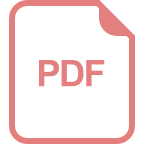
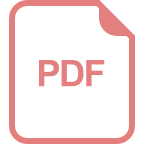












