请用matlab环境,以孪生神经网络为模型框架,写一个指纹匹配模型,要求可以辨别是否为同一指纹
时间: 2024-05-13 21:17:13 浏览: 192
好的,以下是一个简单的孪生神经网络指纹匹配模型的代码示例:
首先,我们需要加载一些指纹图像数据用于训练和测试。这里我们使用了FVC2002的指纹图像数据集。
```matlab
% Load fingerprint images
dataDir = 'path/to/fingerprint/dataset';
imds = imageDatastore(dataDir, 'IncludeSubfolders', true, 'LabelSource', 'foldernames');
```
接下来,我们需要对图像进行预处理,以便将它们输入到神经网络中。我们可以使用Matlab中的图像增强工具箱来进行预处理,例如对图像进行裁剪、旋转、缩放等操作。
```matlab
% Perform image preprocessing
imageSize = [224 224];
augmenter = imageDataAugmenter(...
'RandXReflection', true, ...
'RandRotation', [-20 20], ...
'RandScale', [0.5 1.5], ...
'FillValue', 0);
augimds = augmentedImageDatastore(imageSize, imds, 'DataAugmentation', augmenter);
```
然后,我们可以创建我们的孪生神经网络模型。在这个模型中,我们将使用两个相同的卷积神经网络分别处理两个输入指纹图像,并将它们的输出特征向量送入一个距离度量层来计算它们之间的相似度。
```matlab
% Create the siamese network model
baseNetwork = resnet50;
imageSize = [224 224 3];
input1 = inputLayer(imageSize, 'Name', 'input1');
input2 = inputLayer(imageSize, 'Name', 'input2');
feature1 = baseNetwork(input1);
feature2 = baseNetwork(input2);
l2NormLayer = L2Normalization('norm', 'l2', 'Name', 'l2norm');
distanceLayer = Distance('Name', 'distance');
distance = distanceLayer(feature1, feature2);
similarity = l2NormLayer(distance);
model = dlnetwork(layerGraph([input1 input2 feature1 feature2 distance similarity]));
```
在训练模型之前,我们需要定义损失函数。在孪生神经网络中,通常使用对比损失函数来训练模型。对于每对输入图像,我们将计算它们之间的距离,并将这个距离作为损失函数的一部分。如果这对图像是同一指纹,我们希望距离越小越好;如果它们不是同一指纹,我们希望距离越大越好。
```matlab
% Define the loss function
contrastiveLoss = @(Y, T) contrastiveLossFunction(Y, T);
function loss = contrastiveLossFunction(Y, T)
margin = 1;
D = Y .* T;
loss = (1 - D) * 0.5;
loss(D >= margin) = 0;
loss = mean(loss);
end
```
最后,我们可以使用Adam优化器来训练模型。
```matlab
% Train the model
numEpochs = 10;
miniBatchSize = 16;
initialLearnRate = 0.001;
learnRateDropFactor = 0.1;
learnRateDropPeriod = 5;
l2Reg = 0.0001;
executionEnvironment = "auto";
plots = "training-progress";
options = trainingOptions('adam', ...
'InitialLearnRate', initialLearnRate, ...
'LearnRateDropFactor', learnRateDropFactor, ...
'LearnRateDropPeriod', learnRateDropPeriod, ...
'L2Regularization', l2Reg, ...
'MaxEpochs', numEpochs, ...
'MiniBatchSize', miniBatchSize, ...
'Shuffle', 'every-epoch', ...
'Plots', plots, ...
'Verbose', true, ...
'ExecutionEnvironment', executionEnvironment);
model = trainNetwork(augimds, contrastiveLoss, options);
```
训练完成后,我们可以使用测试数据集来评估模型的性能。
```matlab
% Evaluate the model
testDataDir = 'path/to/test/data';
testImds = imageDatastore(testDataDir, 'IncludeSubfolders', true, 'LabelSource', 'foldernames');
testAugimds = augmentedImageDatastore(imageSize, testImds, 'DataAugmentation', augmenter);
[testLabels, scores] = classify(model, testAugimds);
accuracy = mean(testLabels == testImds.Labels);
fprintf("Accuracy: %.2f%%\n", accuracy * 100);
```
这就是一个简单的孪生神经网络指纹匹配模型的实现。当然,你可以根据自己的需求对模型进行调整和优化。
阅读全文
相关推荐
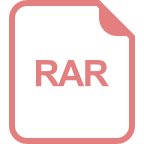
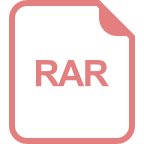
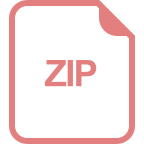
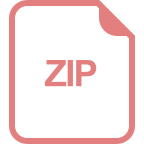
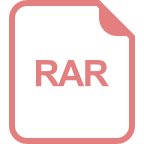
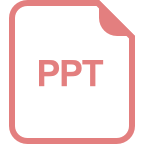
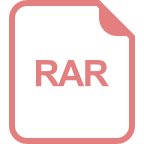
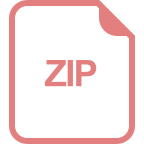
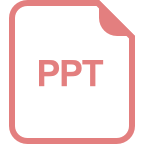
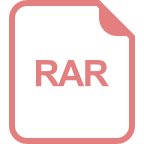
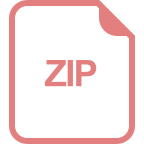
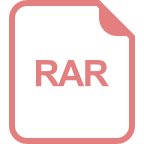

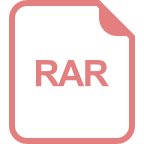