int comp(const void *a,const void *b) { const int *p=a; const int *q=b; return *p - *q; } 改正这段代码的[Error] invalid conversion from 'const void*' to 'const int*' [-fpermissive]错误
时间: 2023-08-30 17:06:04 浏览: 186
将原代码中的 `const int *p=a;` 和 `const int *q=b;` 改为 `const int *p=static_cast<const int*>(a);` 和 `const int *q=static_cast<const int*>(b);` 即可解决这个错误。修改后的代码如下:
```
int comp(const void *a,const void *b) {
const int *p=static_cast<const int*>(a);
const int *q=static_cast<const int*>(b);
return *p - *q;
}
```
这里使用静态转换 (static_cast) 将 `void*` 类型的指针转换为 `const int*` 类型的指针,以消除编译器对指针类型转换的警告和错误。
相关问题
这个代码的意思int comp(const void* a,const void* b) { return *(int*)b - *(int*)a; }
这段代码是一个用于快速排序的比较函数,它的作用是比较两个整数的大小并返回它们的差值。在快速排序中,根据这个差值的正负来判断两个元素的相对位置,从而实现排序。其中,参数a和b是指向待比较元素的指针,使用了void指针来实现通用性。在函数内部,首先将指针转换为int类型的指针,然后再通过解引用操作获取指针所指向的整数值,最后将这两个整数值相减得到它们的差值。如果b所指向的整数大于a所指向的整数,则返回正值,否则返回负值。
帮我用c语言写一段代码,要求如下: Compare the character sequence (CS) of s1 and s2 by dictionary order; 函数名为int comp_chars;形式参数为const char *s1, Uint len1, const char *s2, Uint len2
好的,这是你要求的代码:
```
int comp_chars(const char *s1, Uint len1, const char *s2, Uint len2) {
for (Uint i = 0; i < len1 && i < len2; ++i) {
if (s1[i] < s2[i]) {
return -1;
} else if (s1[i] > s2[i]) {
return 1;
}
}
if (len1 < len2) {
return -1;
} else if (len1 > len2) {
return 1;
} else {
return 0;
}
}
```
这个函数比较两个字符串 s1 和 s2 的字典序。如果 s1 在字典中的顺序更靠前,函数返回 -1;如果 s2 在字典中的顺序更靠前,函数返回 1;如果 s1 和 s2 的字典序相同,函数返回 0。
阅读全文
相关推荐
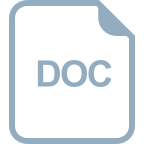














