用循环方法在二叉搜索树插入一个结点
时间: 2023-06-01 07:05:47 浏览: 69
非常感谢您对ChitGPT的关注和提问。关于您的问题,以下是回答:
可以使用以下循环方法在二叉搜索树中插入一个结点:
1. 如果树为空,将该结点作为根节点。
2. 如果树不为空,将该结点从根节点开始与每个结点进行比较。
3. 如果该结点的值小于当前结点的值,则向左子树进行比较。
4. 如果该结点的值大于当前结点的值,则向右子树进行比较。
5. 如果找到一个空结点,则将该结点插入到该空结点的位置。
希望能够帮助到您。如果您还有其他问题,可以继续提问。谢谢!
相关问题
代码举例并详细解释用循环方法在二叉搜索树插入一个结点
好的,下面是代码举例并详细解释使用循环方法在二叉搜索树插入一个结点的过程:
```python
class Node:
def __init__(self, val=None):
self.val = val
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, val):
new_node = Node(val)
if not self.root:
self.root = new_node
return
current_node = self.root
while current_node:
if val < current_node.val:
if current_node.left is None:
current_node.left = new_node
return
current_node = current_node.left
else:
if current_node.right is None:
current_node.right = new_node
return
current_node = current_node.right
```
这里首先定义了节点类 `Node` 和二叉搜索树类 `BinarySearchTree`,然后在 `insert` 方法中实现了在二叉搜索树中插入一个结点的功能。
具体流程如下:
1. 首先创建一个新节点 `new_node`,存储要插入的值;
2. 判断当前二叉搜索树是否为空,如果为空,则将该节点作为树的根节点;
3. 如果树不为空,则从树的根节点开始遍历;
4. 如果 `val` 小于遍历到的当前节点 `current_node` 的值,则向左遍历,如果 `current_node` 的左子树为空,则将 `new_node` 插入到 `current_node` 的左子树中,并返回;
5. 如果 `val` 大于等于遍历到的当前节点 `current_node` 的值,则向右遍历,如果 `current_node` 的右子树为空,则将 `new_node` 插入到 `current_node` 的右子树中,并返回;
6. 如果还没有插入成功,则继续从刚插入的左右子树进行遍历,直到成功插入节点。
这样就可以使用循环方法在二叉搜索树中插入一个结点了。
用用c++实现二叉搜索树(1)从空的二叉树开始,每输入一个节点数据,就建立一个新节点插入到当前已生成的二叉排序树中; (2)在二叉排序树中查找某一结点; (3)删除任一指定的结点并计算查找成功时和查找失败时的平均查找长度
好的,以下是C++实现二叉搜索树的代码:
```c++
#include<iostream>
using namespace std;
struct BSTNode {
int data; //数据域
BSTNode *leftChild; //左子树指针
BSTNode *rightChild; //右子树指针
};
class BSTree {
private:
BSTNode *root; //根节点指针
public:
BSTree() { root = NULL; } //构造函数
void insertNode(int); //插入节点
void searchNode(int); //查找节点
void deleteNode(int); //删除节点
int searchCount(int); //查找节点个数
int getDepth(BSTNode *); //获取深度
void inOrder(BSTNode *); //中序遍历
void preOrder(BSTNode *); //前序遍历
void postOrder(BSTNode *); //后序遍历
};
//插入节点
void BSTree::insertNode(int data) {
BSTNode *newNode = new BSTNode; //创建新节点
newNode->data = data; //赋值
newNode->leftChild = NULL;
newNode->rightChild = NULL;
if (root == NULL) { //如果根节点为空
root = newNode; //将新节点作为根节点
}
else {
BSTNode *current = root;
while (true) { //循环查找插入位置
if (data < current->data) {
if (current->leftChild == NULL) {
current->leftChild = newNode;
break;
}
else {
current = current->leftChild;
}
}
else {
if (current->rightChild == NULL) {
current->rightChild = newNode;
break;
}
else {
current = current->rightChild;
}
}
}
}
}
//查找节点
void BSTree::searchNode(int data) {
BSTNode *current = root;
int count = 0;
while (current != NULL) {
count++;
if (data == current->data) {
cout << "查找成功!查找次数为:" << count << endl;
return;
}
else if (data < current->data) {
current = current->leftChild;
}
else {
current = current->rightChild;
}
}
cout << "查找失败!查找次数为:" << count << endl;
}
//删除节点
void BSTree::deleteNode(int data) {
BSTNode *current = root;
BSTNode *parent = NULL;
while (current != NULL && current->data != data) {
parent = current;
if (data < current->data) {
current = current->leftChild;
}
else {
current = current->rightChild;
}
}
if (current == NULL) {
cout << "该节点不存在!" << endl;
return;
}
if (current->leftChild == NULL && current->rightChild == NULL) { //情况1:该节点为叶节点
if (current == root) {
root = NULL;
}
else {
if (current == parent->leftChild) {
parent->leftChild = NULL;
}
else {
parent->rightChild = NULL;
}
}
delete current;
}
else if (current->leftChild != NULL && current->rightChild == NULL) { //情况2:该节点只有左子树
if (current == root) {
root = current->leftChild;
}
else {
if (current == parent->leftChild) {
parent->leftChild = current->leftChild;
}
else {
parent->rightChild = current->leftChild;
}
}
delete current;
}
else if (current->leftChild == NULL && current->rightChild != NULL) { //情况2:该节点只有右子树
if (current == root) {
root = current->rightChild;
}
else {
if (current == parent->leftChild) {
parent->leftChild = current->rightChild;
}
else {
parent->rightChild = current->rightChild;
}
}
delete current;
}
else { //情况3:该节点既有左子树,又有右子树
BSTNode *successor = current->rightChild;
while (successor->leftChild != NULL) {
successor = successor->leftChild;
}
int temp = successor->data;
deleteNode(successor->data);
current->data = temp;
}
}
//查找节点个数
int BSTree::searchCount(int data) {
BSTNode *current = root;
int count = 0;
while (current != NULL) {
count++;
if (data == current->data) {
return count;
}
else if (data < current->data) {
current = current->leftChild;
}
else {
current = current->rightChild;
}
}
return 0;
}
//获取深度
int BSTree::getDepth(BSTNode *node) {
if (node == NULL) {
return 0;
}
int leftDepth = getDepth(node->leftChild);
int rightDepth = getDepth(node->rightChild);
return leftDepth > rightDepth ? leftDepth + 1 : rightDepth + 1;
}
//中序遍历
void BSTree::inOrder(BSTNode *node) {
if (node != NULL) {
inOrder(node->leftChild);
cout << node->data << " ";
inOrder(node->rightChild);
}
}
//前序遍历
void BSTree::preOrder(BSTNode *node) {
if (node != NULL) {
cout << node->data << " ";
preOrder(node->leftChild);
preOrder(node->rightChild);
}
}
//后序遍历
void BSTree::postOrder(BSTNode *node) {
if (node != NULL) {
postOrder(node->leftChild);
postOrder(node->rightChild);
cout << node->data << " ";
}
}
int main() {
BSTree bst;
//插入节点
bst.insertNode(15);
bst.insertNode(6);
bst.insertNode(18);
bst.insertNode(3);
bst.insertNode(7);
bst.insertNode(17);
bst.insertNode(20);
bst.insertNode(2);
bst.insertNode(4);
bst.insertNode(13);
bst.insertNode(9);
//中序遍历
cout << "中序遍历结果:";
bst.inOrder(bst.root);
cout << endl;
//查找节点
bst.searchNode(13);
bst.searchNode(8);
//删除节点
bst.deleteNode(13);
bst.deleteNode(6);
bst.deleteNode(15);
//中序遍历
cout << "中序遍历结果:";
bst.inOrder(bst.root);
cout << endl;
//前序遍历
cout << "前序遍历结果:";
bst.preOrder(bst.root);
cout << endl;
//后序遍历
cout << "后序遍历结果:";
bst.postOrder(bst.root);
cout << endl;
//查找节点个数
cout << "节点18的查找次数为:" << bst.searchCount(18) << endl;
cout << "节点8的查找次数为:" << bst.searchCount(8) << endl;
//获取深度
cout << "树的深度为:" << bst.getDepth(bst.root) << endl;
return 0;
}
```
在该代码中,我们首先定义了一个二叉搜索树的节点结构 `BSTNode`,包括数据域 `data` 和左右子树指针 `leftChild` 和 `rightChild`。然后定义了一个二叉搜索树类 `BSTree`,包含插入节点、查找节点、删除节点、查找节点个数、获取深度、中序遍历、前序遍历和后序遍历等操作。
我们通过 `insertNode` 方法向二叉搜索树插入节点,通过 `searchNode` 方法查找节点,并统计查找次数,通过 `deleteNode` 方法删除节点,通过 `searchCount` 方法查找节点个数,通过 `getDepth` 方法获取树的深度,通过 `inOrder`、`preOrder` 和 `postOrder` 方法分别进行中序遍历、前序遍历和后序遍历。
在 `main` 函数中,我们创建一个二叉搜索树对象 `bst`,插入了一些节点,并进行一些操作,包括中序遍历、查找节点、删除节点、前序遍历、后序遍历、查找节点个数和获取深度等。
以上就是用 C++ 实现二叉搜索树的完整代码,希望能对你有所帮助。
阅读全文
相关推荐
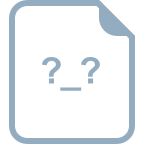
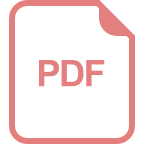
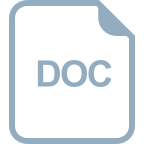


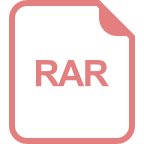
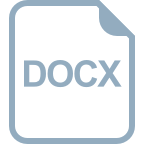
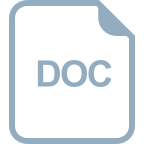
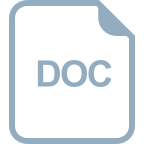
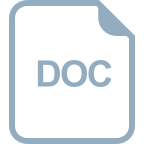
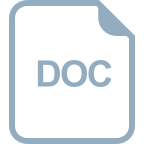
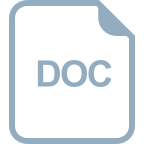
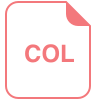

