基于激光雷达点云检测道路可行驶区域(道路边界、不平度,车道线等),给我代码,并说明如何使用
时间: 2024-05-06 20:20:28 浏览: 23
由于代码涉及到激光雷达数据的处理,需要使用相关的激光雷达驱动和点云库,推荐使用ROS(Robot Operating System)作为开发环境。
以下是一个基于ROS和PCL(Point Cloud Library)的激光雷达点云处理代码示例,用于检测道路可行驶区域:
```python
#!/usr/bin/env python
import rospy
from sensor_msgs.msg import PointCloud2
import pcl
class RoadDetection:
def __init__(self):
rospy.init_node('road_detection')
self.pc_sub = rospy.Subscriber('/lidar/point_cloud', PointCloud2, self.callback)
self.pc_pub = rospy.Publisher('/lidar/road_point_cloud', PointCloud2, queue_size=10)
def callback(self, pc_msg):
# Convert ROS PointCloud2 message to PCL PointCloud
pc = pcl.PointCloud.PointXYZI.fromROSMsg(pc_msg)
# Remove ground plane using RANSAC
seg = pc.make_segmenter_normals(ksearch=50)
seg.set_optimize_coefficients(True)
seg.set_model_type(pcl.SACMODEL_PLANE)
seg.set_method_type(pcl.SAC_RANSAC)
seg.set_distance_threshold(0.1)
seg.set_normal_distance_weight(0.1)
indices, model = seg.segment()
cloud_plane = pc.extract(indices, negative=False)
cloud = pc.extract(indices, negative=True)
# Remove outliers using statistical outlier removal
sor = cloud.make_statistical_outlier_filter()
sor.set_mean_k(50)
sor.set_std_dev_mul_thresh(1.0)
cloud = sor.filter()
# Extract road boundaries using Euclidean clustering
tree = cloud.make_kdtree()
ec = cloud.make_EuclideanClusterExtraction()
ec.set_ClusterTolerance(0.5)
ec.set_MinClusterSize(100)
ec.set_MaxClusterSize(10000)
ec.set_SearchMethod(tree)
cluster_indices = ec.Extract()
road_boundaries = pcl.PointCloud.PointXYZI()
for indices in cluster_indices:
cloud_cluster = cloud.extract(indices)
road_boundaries += cloud_cluster
# Publish the road boundaries point cloud
self.pc_pub.publish(road_boundaries.toROSMsg())
if __name__ == '__main__':
rd = RoadDetection()
rospy.spin()
```
代码中使用了ROS的消息订阅和发布机制,订阅了/lidar/point_cloud主题的激光雷达点云数据,并通过ROS的消息发布机制将检测到的道路边界点云数据发布到/lidar/road_point_cloud主题中。
使用方法:
1. 安装ROS和PCL库
2. 创建ROS工作空间
3. 将代码保存到工作空间的src目录下,编译ROS包
4. 启动ROS节点
```
roscore
```
5. 运行代码
```
rosrun <package_name> road_detection.py
```
6. 查看道路边界点云数据
```
rviz
```
在rviz中添加PointCloud2类型的可视化对象,选择订阅/lidar/road_point_cloud主题,即可显示道路边界点云数据。
相关推荐
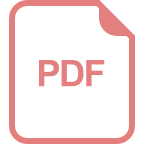
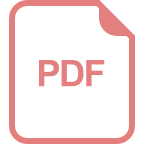
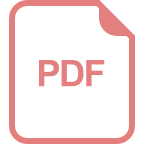














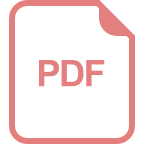