爬取当当网图书的评论 如何利用bs4写代码
时间: 2024-03-29 07:36:25 浏览: 13
可以使用Python的Requests和BeautifulSoup库来爬取当当网图书的评论。以下是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 当当网图书详情页面的URL
url = "http://product.dangdang.com/12345678.html"
# 发送HTTP GET请求获取HTML页面
response = requests.get(url)
# 使用BeautifulSoup解析HTML页面
soup = BeautifulSoup(response.text, "html.parser")
# 获取评论列表
comments = soup.find_all("div", class_="comment_item")
# 遍历评论列表并输出评论内容
for comment in comments:
content = comment.find("div", class_="describe_detail").text.strip()
print(content)
```
请将上面的代码中的`url`替换为你要爬取的当当网图书详情页的URL,然后运行代码即可获取评论内容。注意,由于当当网有反爬虫措施,可能需要使用代理IP或者延时等方式来避免被封IP。
相关问题
爬取当当网书籍评论用Python代码
以下是使用 Python 代码爬取当当网书籍评论的示例程序:
```python
import requests
from bs4 import BeautifulSoup
# 设置请求头
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 设置要爬取的书籍 ID
book_id = '12345678'
# 设置要爬取的评论页数
page_num = 3
# 循环爬取每一页的评论
for page in range(1, page_num + 1):
# 构造评论页面的 URL
url = f'http://product.dangdang.com/index.php?r=comment%2Flist&productId={book_id}&categoryPath=01.00.00.00.00.00&pageIndex={page}&sortType=1'
# 发送 GET 请求
response = requests.get(url, headers=headers)
# 解析 HTML
soup = BeautifulSoup(response.text, 'html.parser')
# 找到所有评论项
items = soup.find_all('div', {'class': 'comment_items'})
# 遍历每一个评论项
for item in items:
# 找到评论内容
content = item.find('div', {'class': 'describe_detail'}).text.strip()
# 找到评论时间
time = item.find('span', {'class': 'time'}).text.strip()
# 打印评论内容和时间
print(content)
print(time)
```
以上代码使用 requests 库发送 GET 请求,使用 BeautifulSoup 库解析 HTML,找到每个评论项并提取评论内容和时间。可以根据实际需要修改代码中的书籍 ID 和评论页数。
python爬取当当网书籍评论
要爬取当当网书籍评论,可以使用 Python 的 requests 库和 BeautifulSoup 库。以下是一个简单的爬取当当网某本书籍评论的示例代码:
```
import requests
from bs4 import BeautifulSoup
book_id = '123456' # 替换成你要爬取的书籍ID
url = f'https://product.dangdang.com/{book_id}.html'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
comment_list = soup.find_all('div', {'class': 'comment_items clearfix'})
for comment in comment_list:
user = comment.find('div', {'class': 'name'}).text.strip()
rating = comment.find('span', {'class': 'star'}).get('class')[1][-1]
content = comment.find('div', {'class': 'describe_detail'}).text.strip()
print(f'用户:{user},评分:{rating}星,评论:{content}')
```
注意,当当网有反爬机制,如果频繁爬取可能会被封 IP。建议加入适当的延时和代理池等措施。另外,爬取数据需要遵守相关法律法规和网站规定,不得用于商业用途。
相关推荐
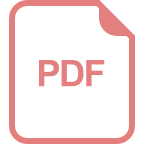
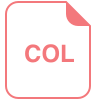
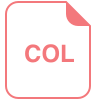
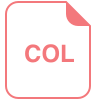
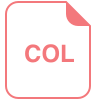








