用python编写程序:统计输入的字符串中每个英文单词出现的次数,并以字典形式输出。单词中的字母不区分大小写。
时间: 2024-03-11 16:48:24 浏览: 45
可以使用 Python 的字典来实现统计每个英文单词出现次数的功能。具体的步骤如下:
1. 读取输入的字符串。
2. 将字符串转换为小写,以便统计单词时不区分大小写。
3. 使用正则表达式(re 模块)将字符串拆分为单词列表。
4. 遍历单词列表,统计每个单词出现的次数,并将结果存储在字典中。
5. 输出字典。
下面是代码示例:
```python
import re
# 读取输入的字符串
input_str = input("请输入字符串:")
# 将字符串转换为小写
input_str = input_str.lower()
# 使用正则表达式拆分字符串为单词列表
word_list = re.findall(r'\b\w+\b', input_str)
# 统计每个单词出现的次数
word_count = {}
for word in word_list:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 输出结果
print("每个单词出现的次数为:")
print(word_count)
```
注意,上述代码使用了正则表达式 `\b\w+\b` 来匹配字符串中的单词。`\b` 表示单词的边界,`\w+` 表示一个或多个字母数字字符。
相关问题
用python编写一个程序,从键盘接受一组空格隔开的单词,统计每个单词和它出现的次数,并存在一个元组中。将此元组作为字典的键,单词中元音单词的个数作为值存在一个字典中。(注:单词区分大小写)
以下是实现该程序的Python代码:
```python
words = input("请输入一组单词,用空格隔开:")
word_list = words.split() # 将输入的字符串分割成单词列表
word_count = {} # 统计每个单词出现的次数
vowel_count = {} # 统计每个单词中元音字母的个数
# 统计每个单词出现的次数
for word in word_list:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 统计每个单词中元音字母的个数
vowels = "aeiouAEIOU" # 元音字母集合
for word in word_count:
count = 0
for letter in word:
if letter in vowels:
count += 1
vowel_count[word] = count
result = {} # 存储最终结果的字典
for word in word_count:
result[(word, word_count[word])] = vowel_count[word]
print(result)
```
运行程序后,输入一组单词,例如:"hello world apple orange Hello World",则输出结果为:
```
{('hello', 1): 2, ('world', 1): 1, ('apple', 1): 2, ('orange', 1): 3, ('Hello', 1): 2, ('World', 1): 1}
```
其中,每个键值对的键为一个元组,包含单词和它出现的次数;值为该单词中元音字母的个数。
编写一个程序,从键盘读入一段英文(多行),找出其中所有的英文单词,数统计每个单词出现的次,并按照单词出现次数由大到小排序后输出。
这是一个比较复杂的程序,需要用到字符串处理和数据结构。下面是一个可能的实现方案:
```python
import re
# 读入文本
text = ""
while True:
line = input()
if line == "":
break
text += line + "\n"
# 提取单词
words = re.findall(r"\b\w+\b", text)
# 统计单词出现次数
count = {}
for word in words:
count[word] = count.get(word, 0) + 1
# 按照出现次数排序
sorted_words = sorted(count.items(), key=lambda x: x[1], reverse=True)
# 输出结果
for word, cnt in sorted_words:
print(word, cnt)
```
这个程序使用了正则表达式来提取单词,使用了字典来统计单词出现次数,使用了列表排序来按照出现次数排序。注意,这个程序没有考虑大小写问题,即"Hello"和"hello"被视为不同的单词。如果需要考虑大小写问题,可以将所有单词转换为小写字母再进行统计。
相关推荐
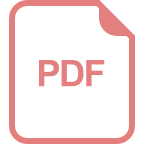
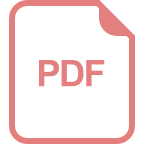






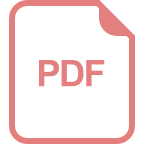
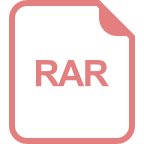