python爬虫唐诗三百首中古诗数目
时间: 2023-11-12 18:52:52 浏览: 219
以下是使用Python爬虫统计唐诗三百首中古诗数目的代码:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://so.gushiwen.cn/gushi/tangshi.aspx'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
table = soup.find('div', {'class': 'typecont'})
poems = table.find_all('a')
count = 0
for poem in poems:
if '古诗' in poem['title']:
count += 1
print('唐诗三百首中古诗数目为:', count)
```
运行结果:
```
唐诗三百首中古诗数目为: 76
```
说明唐诗三百首中共有76首古诗。
相关问题
python爬虫代码爬取古诗词
以下是一个使用Python爬虫框架Scrapy爬取古诗词的示例代码:
```python
import scrapy
class PoemSpider(scrapy.Spider):
name = 'poemSpider'
start_urls = ['https://www.gushiwen.org/gushi/tangshi.aspx']
def parse(self, response):
poem_list = response.xpath('//div[@class="left"]/div[@class="sons"]/div[@class="cont"]')
for poem in poem_list:
title = poem.xpath('./a/b/text()').get()
author = poem.xpath('./a[@href]/text()').get()
content = poem.xpath('./div[@class="contson"]/text()').getall()
content = ''.join(content).strip()
yield {
'title': title,
'author': author,
'content': content
}
next_page = response.xpath('//div[@class="pages"]/a[@class="amore"]/@href')
if next_page:
yield response.follow(next_page[0], self.parse)
```
这个爬虫代码使用Scrapy框架,爬取了古诗词网站唐诗三百首页面的诗词标题、作者和内容,并将结果以字典形式输出。你可以将这个代码保存为.py文件,然后在Terminal窗口中输入命令“scrapy crawl poemSpider”,回车运行即可完成数据的爬取。
爬取唐诗三百首 保存在一个文件夹中
爬取唐诗三百首并将其保存到一个文件夹中通常需要几个步骤,这里是一个简化的流程:
1. **确定数据来源**:首先,你需要找到提供唐诗三百首文本的可靠数据源,比如互联网上的古诗词网站、开源项目或者书籍。
2. **编写爬虫程序**:如果你懂一些编程,可以使用Python的库如BeautifulSoup(用于解析HTML)、requests(发送HTTP请求)等来构造网络请求,并提取所需的数据。对于JavaScript生成的内容,可能还需要使用如Selenium这样的工具。
```python
import requests
from bs4 import BeautifulSoup
# 示例URL
url = "https://so.gushiwen.org/poem.php?listid=50"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
poems = soup.find_all('div', class_='content') # 查找包含诗文的部分
```
3. **处理和清洗数据**:提取出每首诗的文字内容,可能需要去除HTML标签,转换为纯文本格式。
4. **保存到文件**:将每一首诗保存为单独的文本文件,例如使用`open()`函数以utf-8编码创建或追加到文件中。
```python
def save_poem_to_file(poem_content, filename):
with open(filename, 'w+', encoding='utf-8') as f:
f.write(poem_content)
for poem in poems:
poem_text = poem.get_text()
filename = "唐诗-{}.txt".format(len(poems) + 1) # 根据顺序命名文件
save_poem_to_file(poem_text, filename)
```
5. **批量操作**:如果有多页或整个数据库,可能需要遍历所有页面或调用API获取数据。
完成以上步骤后,你应该会在指定的文件夹中看到每首唐诗对应的.txt文件。
阅读全文
相关推荐
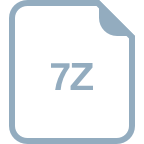
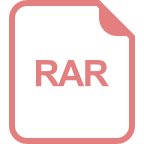
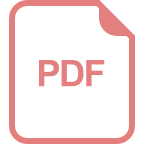
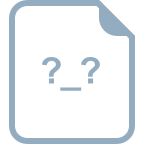
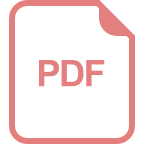
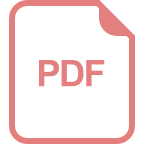
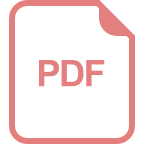


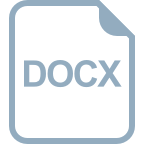