基于遗传算法改进的BP神经网络算法代码
时间: 2023-09-07 11:17:38 浏览: 113
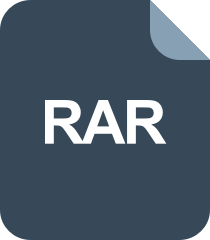
基于遗传算法的BP神经网络优化算法
以下是基于遗传算法改进的BP神经网络算法的Python实现代码:
```python
import numpy as np
class BPNN_GA:
def __init__(self, input_size, hidden_size, output_size):
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
# 初始化网络参数
self.W1 = np.random.randn(self.input_size, self.hidden_size)
self.b1 = np.random.randn(self.hidden_size)
self.W2 = np.random.randn(self.hidden_size, self.output_size)
self.b2 = np.random.randn(self.output_size)
# 设置遗传算法参数
self.pop_size = 100
self.max_iter = 100
self.mutation_rate = 0.01
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def forward(self, X):
# 前向传播计算输出结果
h = np.dot(X, self.W1) + self.b1
h = self.sigmoid(h)
y = np.dot(h, self.W2) + self.b2
return y
def loss(self, X, y_true):
# 计算均方误差损失
y_pred = self.forward(X)
loss = np.mean((y_true - y_pred) ** 2)
return loss
def fit(self, X, y):
# 遗传算法优化BP神经网络参数
pop = self.init_pop()
for i in range(self.max_iter):
pop = self.select(pop, X, y)
pop = self.crossover(pop)
pop = self.mutate(pop)
# 使用最优个体更新网络参数
self.update_params(pop[0])
def init_pop(self):
# 初始化种群
pop = []
for i in range(self.pop_size):
W1 = np.random.randn(self.input_size, self.hidden_size)
b1 = np.random.randn(self.hidden_size)
W2 = np.random.randn(self.hidden_size, self.output_size)
b2 = np.random.randn(self.output_size)
pop.append((W1, b1, W2, b2))
return pop
def select(self, pop, X, y):
# 选择适应度高的个体
fitness = []
for i in range(self.pop_size):
W1, b1, W2, b2 = pop[i]
self.update_params((W1, b1, W2, b2))
loss = self.loss(X, y)
fitness.append((i, loss))
fitness.sort(key=lambda x: x[1])
pop = [pop[i[0]] for i in fitness[:int(self.pop_size/2)]]
return pop
def crossover(self, pop):
# 交叉产生新个体
new_pop = []
for i in range(self.pop_size):
parent1 = pop[np.random.randint(0, len(pop))]
parent2 = pop[np.random.randint(0, len(pop))]
W1 = np.where(np.random.rand(*parent1[0].shape) < 0.5,
parent1[0], parent2[0])
b1 = np.where(np.random.rand(*parent1[1].shape) < 0.5,
parent1[1], parent2[1])
W2 = np.where(np.random.rand(*parent1[2].shape) < 0.5,
parent1[2], parent2[2])
b2 = np.where(np.random.rand(*parent1[3].shape) < 0.5,
parent1[3], parent2[3])
new_pop.append((W1, b1, W2, b2))
return new_pop
def mutate(self, pop):
# 突变产生新个体
new_pop = []
for i in range(self.pop_size):
W1, b1, W2, b2 = pop[i]
W1 = np.where(np.random.rand(*W1.shape) < self.mutation_rate,
np.random.randn(*W1.shape), W1)
b1 = np.where(np.random.rand(*b1.shape) < self.mutation_rate,
np.random.randn(*b1.shape), b1)
W2 = np.where(np.random.rand(*W2.shape) < self.mutation_rate,
np.random.randn(*W2.shape), W2)
b2 = np.where(np.random.rand(*b2.shape) < self.mutation_rate,
np.random.randn(*b2.shape), b2)
new_pop.append((W1, b1, W2, b2))
return new_pop
def update_params(self, params):
# 更新网络参数
self.W1, self.b1, self.W2, self.b2 = params
def predict(self, X):
# 生成预测结果
y_pred = self.forward(X)
return y_pred
```
在这个实现中,我们使用了遗传算法来优化BP神经网络的参数。我们使用均方误差损失作为适应度函数,选择适应度高的个体进行交叉和突变产生新个体。最后,我们使用最优个体的参数来更新网络。
阅读全文
相关推荐
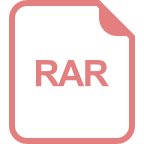
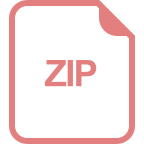
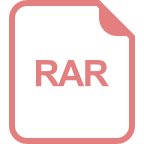
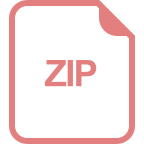
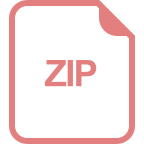
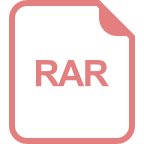
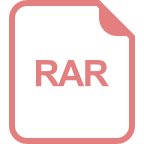
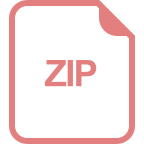
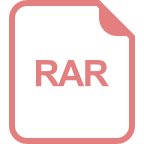
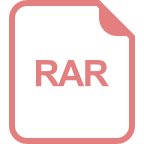
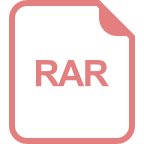
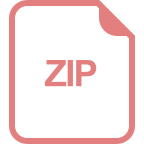
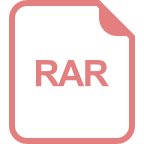
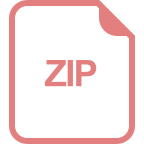
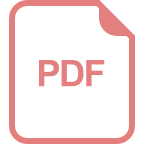
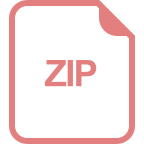
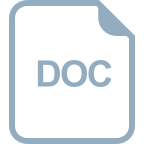