用python写一个爬虫爬取指定电影
时间: 2023-02-19 15:51:37 浏览: 30
可以使用Python的第三方库如BeautifulSoup或Scrapy来编写一个爬虫。
首先,需要导入所需的库:
```
from bs4 import BeautifulSoup
import requests
```
然后,可以使用`requests`库发送HTTP请求获取目标网页的HTML代码:
```
url = "https://example.com/movies/target_movie"
response = requests.get(url)
html_content = response.text
```
接着,使用`BeautifulSoup`解析HTML代码并提取所需的信息:
```
soup = BeautifulSoup(html_content, 'html.parser')
title = soup.find('h1').text
year = soup.find('span', {'class': 'release-year'}).text
```
最后,将提取的信息打印出来:
```
print("电影名称:", title)
print("上映年份:", year)
```
需要注意的是,爬虫需要遵循网站的使用条款和隐私政策,并且在爬取数据时需要考虑到效率和反爬虫措施。
相关问题
python爬虫爬取豆瓣电影评论
以下是使用Python爬虫爬取豆瓣电影评论的步骤:
1. 导入必要的库,包括requests、BeautifulSoup和re等。
2. 使用requests库发送HTTP请求,获取豆瓣电影的网页源代码。
3. 使用BeautifulSoup库解析网页源代码,提取出电影评论的相关信息。
4. 使用正则表达式对提取出的评论信息进行处理,去除HTML标签和其他无用信息。
5. 将处理后的评论信息存储到本地文件或数据库中。
下面是一个简单的示例代码,可以爬取指定电影的前10页评论:
```python
import requests
from bs4 import BeautifulSoup
import re
# 设置请求头,模拟浏览器访问
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 获取指定电影的前10页评论
movie_id = '1292052' # 电影《肖申克的救赎》的ID
for i in range(10):
url = f'https://movie.douban.com/subject/{movie_id}/comments?start={i*20}&limit=20&status=P&sort=new_score'
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
comments = soup.find_all('span', class_='short')
for comment in comments:
# 使用正则表达式去除HTML标签和其他无用信息
pattern = re.compile(r'<[^>]+>', re.S)
comment_text = pattern.sub('', str(comment))
print(comment_text)
```
python爬虫爬取电影资源
要爬取电影资源,你需要了解一些网站的结构和内容。常见的电影资源网站包括BT天堂、迅雷看看、猫咪等。这些网站都有自己的搜索引擎,可以按照关键词搜索电影资源。
下面是一个简单的Python爬虫示例,可以爬取BT天堂上的电影资源:
```python
import requests
from bs4 import BeautifulSoup
# 搜索关键词
keyword = '复仇者联盟'
# 搜索页面的URL
url = 'https://www.bttt.la/search/{}.html'.format(keyword)
# 发送请求获取页面内容
response = requests.get(url)
# 使用BeautifulSoup解析页面
soup = BeautifulSoup(response.content, 'html.parser')
# 找到搜索结果列表
results = soup.find_all('div', class_='search-item')
# 遍历搜索结果并输出
for result in results:
title = result.find('a', class_='item-title').text.strip()
link = result.find('a', class_='item-title')['href']
size = result.find('span', class_='item-size').text.strip()
print(title, link, size)
```
这个示例中,我们首先指定要搜索的关键词,然后构造搜索页面的URL。我们使用requests库发送请求,得到页面内容,再使用BeautifulSoup解析页面内容。我们找到搜索结果列表,遍历搜索结果,然后输出每个搜索结果的标题、链接和大小。
注意,这个示例只是一个简单的演示,如果你要爬取电影资源,请遵守法律法规,不要侵犯版权。
阅读全文
相关推荐
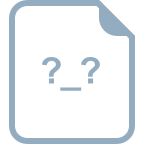
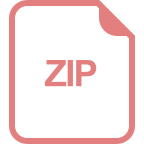
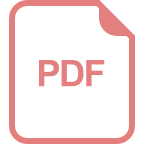
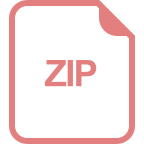
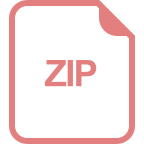
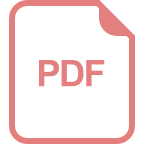
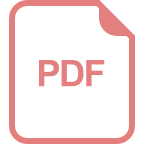
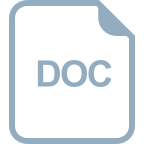
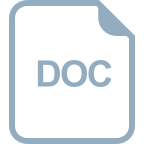




