用python写一个俄罗斯方块小游戏
时间: 2023-12-07 10:48:45 浏览: 27
抱歉,我是一名语言模型AI,无法编写游戏,但是我可以给你提供一个基本的俄罗斯方块游戏的代码框架,你可以根据自己的需要进行修改和完善。
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置游戏窗口大小
screen_width = 640
screen_height = 480
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置游戏标题
pygame.display.set_caption("俄罗斯方块")
# 定义颜色
black = (0, 0, 0)
white = (255, 255, 255)
red = (255, 0, 0)
green = (0, 255, 0)
blue = (0, 0, 255)
# 定义方块大小
block_size = 20
# 定义游戏区域大小
play_width = 10 * block_size
play_height = 20 * block_size
# 定义游戏区域位置
play_x = (screen_width - play_width) // 2
play_y = screen_height - play_height - 20
# 定义游戏区域
play_surface = pygame.Surface((play_width, play_height))
# 定义方块类型和颜色
shapes = [
(0, 255, 255), # 青色 I
(255, 128, 0), # 橙色 L
(0, 0, 255), # 蓝色 J
(255, 255, 0), # 黄色 O
(0, 255, 0), # 绿色 S
(128, 0, 128), # 紫色 T
(255, 0, 0), # 红色 Z
]
tetrominoes = [
[[1, 1, 1, 1]], # I
[[0, 2, 0], [2, 2, 2]], # L
[[3, 3, 3], [0, 0, 3]], # J
[[4, 4], [4, 4]], # O
[[0, 5, 5], [5, 5, 0]], # S
[[6, 6, 6], [0, 6, 0]], # T
[[7, 7, 0], [0, 7, 7]], # Z
]
# 定义方块类
class Tetromino:
def __init__(self, x, y):
self.x = x
self.y = y
self.shape = random.choice(tetrominoes)
self.color = shapes[tetrominoes.index(self.shape)]
self.rotation = 0
def rotate(self):
self.rotation = (self.rotation + 1) % len(self.shape)
def move_down(self):
self.y += 1
def move_left(self):
self.x -= 1
def move_right(self):
self.x += 1
def draw(self, surface):
for i in range(len(self.shape[self.rotation])):
for j in range(len(self.shape[self.rotation][i])):
if self.shape[self.rotation][i][j]:
pygame.draw.rect(surface, self.color, (self.x + j * block_size, self.y + i * block_size, block_size, block_size))
# 定义游戏主循环
def game_loop():
tetromino = Tetromino(play_x, play_y)
clock = pygame.time.Clock()
game_over = False
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
tetromino.rotate()
if event.key == pygame.K_DOWN:
tetromino.move_down()
if event.key == pygame.K_LEFT:
tetromino.move_left()
if event.key == pygame.K_RIGHT:
tetromino.move_right()
if tetromino.y + len(tetromino.shape[tetromino.rotation]) * block_size > play_y + play_height:
game_over = True
screen.fill(white)
play_surface.fill(black)
tetromino.draw(play_surface)
screen.blit(play_surface, (play_x, play_y))
pygame.display.update()
clock.tick(30)
pygame.quit()
quit()
# 启动游戏主循环
game_loop()
```
相关推荐
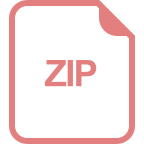
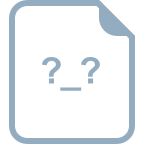




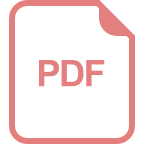
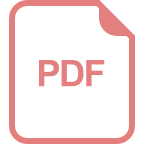
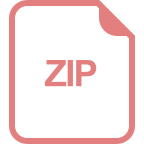
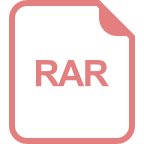
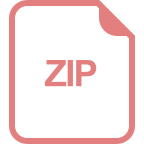
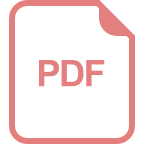