用tensorflow写一段多尺度卷积神经网络的代码
时间: 2023-11-13 19:04:25 浏览: 71
以下是使用TensorFlow编写的多尺度卷积神经网络的示例代码:
```python
import tensorflow as tf
# 定义输入数据的占位符
x = tf.placeholder(tf.float32, [None, 32, 32, 3])
y_true = tf.placeholder(tf.float32, [None, 10])
# 定义卷积层的函数
def conv2d(x, filters, kernel_size, stride, padding='SAME', activation=tf.nn.relu):
conv = tf.layers.conv2d(x, filters, kernel_size, stride, padding, activation=activation)
return conv
# 定义多尺度卷积神经网络
def multiscale_cnn(x):
# 第一层卷积
conv1_3x3 = conv2d(x, 64, [3, 3], 1)
conv1_5x5 = conv2d(x, 64, [5, 5], 1)
conv1_7x7 = conv2d(x, 64, [7, 7], 1)
pool1 = tf.layers.max_pooling2d(conv1_3x3 + conv1_5x5 + conv1_7x7, [2, 2], [2, 2], 'SAME')
# 第二层卷积
conv2_3x3 = conv2d(pool1, 128, [3, 3], 1)
conv2_5x5 = conv2d(pool1, 128, [5, 5], 1)
conv2_7x7 = conv2d(pool1, 128, [7, 7], 1)
pool2 = tf.layers.max_pooling2d(conv2_3x3 + conv2_5x5 + conv2_7x7, [2, 2], [2, 2], 'SAME')
# 第三层卷积
conv3_3x3 = conv2d(pool2, 256, [3, 3], 1)
conv3_5x5 = conv2d(pool2, 256, [5, 5], 1)
conv3_7x7 = conv2d(pool2, 256, [7, 7], 1)
pool3 = tf.layers.max_pooling2d(conv3_3x3 + conv3_5x5 + conv3_7x7, [2, 2], [2, 2], 'SAME')
# 展开特征图
flatten = tf.layers.flatten(pool3)
# 全连接层
fc1 = tf.layers.dense(flatten, 1024, activation=tf.nn.relu)
fc2 = tf.layers.dense(fc1, 512, activation=tf.nn.relu)
logits = tf.layers.dense(fc2, 10)
return logits
# 构建模型
logits = multiscale_cnn(x)
# 定义损失函数和优化器
cross_entropy = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(labels=y_true, logits=logits))
train_op = tf.train.AdamOptimizer(1e-4).minimize(cross_entropy)
# 定义准确率
correct_prediction = tf.equal(tf.argmax(logits, 1), tf.argmax(y_true, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
# 训练模型
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for i in range(NUM_EPOCHS):
for j in range(NUM_BATCHES):
batch_x, batch_y = get_batch(train_data, BATCH_SIZE)
sess.run(train_op, feed_dict={x: batch_x, y_true: batch_y})
val_acc = sess.run(accuracy, feed_dict={x: val_data, y_true: val_labels})
print("Epoch:", i+1, "Validation Accuracy:", val_acc)
```
此示例中,我们定义了一个三层卷积神经网络,每层卷积使用不同大小的卷积核(3x3、5x5和7x7)来提取不同尺度的特征。在每个卷积层之后,我们使用最大池化层来缩小特征图的大小。最后,我们将特征图展开并传递到全连接层,得出输出。我们使用softmax交叉熵作为损失函数,并使用Adam优化器进行优化。我们还计算了准确率,以评估模型在验证集上的表现。
相关推荐
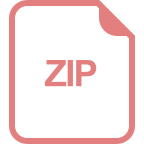
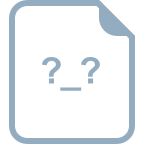














