pytorch用数据和物理信息驱动方法求解PDE间断问题的代码
时间: 2023-11-28 18:51:30 浏览: 44
以下是一个使用 PyTorch 实现数据驱动方法求解 PDE 间断问题的代码示例:
```python
import torch
import numpy as np
from torch.autograd import grad
import matplotlib.pyplot as plt
# 定义 PDE 的右边函数
def f(u):
return torch.exp(-u)
# 定义 PDE 的初值
def u0(x):
return torch.sin(np.pi*x)
# 定义模型
class Net(torch.nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = torch.nn.Linear(1, 10)
self.fc2 = torch.nn.Linear(10, 10)
self.fc3 = torch.nn.Linear(10, 1)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.relu(self.fc2(x))
x = self.fc3(x)
return x
# 定义训练函数
def train(model, optimizer, x_train, y_train, epochs):
for epoch in range(epochs):
optimizer.zero_grad()
outputs = model(x_train)
loss = torch.mean((grad(outputs, x_train, create_graph=True)[0] - f(outputs))**2) + torch.mean((outputs[0] - u0(x_train[0]))**2)
loss.backward()
optimizer.step()
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, epochs, loss.item()))
# 定义测试函数
def test(model, x_test):
outputs = model(x_test)
plt.plot(x_test.detach().numpy(), outputs.detach().numpy(), label='Prediction')
plt.plot(x_test.detach().numpy(), u0(x_test).detach().numpy(), label='Exact')
plt.legend()
plt.show()
# 生成训练数据
x_train = torch.linspace(0, 1, 100).reshape(-1, 1)
y_train = u0(x_train)
# 初始化模型和优化器
model = Net()
optimizer = torch.optim.Adam(model.parameters(), lr=0.01)
# 训练模型
train(model, optimizer, x_train, y_train, epochs=1000)
# 测试模型
x_test = torch.linspace(0, 1, 100).reshape(-1, 1)
test(model, x_test)
```
在上面的代码中,我们定义了一个简单的神经网络模型 `Net`,它有三个全连接层,每个层都使用 ReLU 激活函数。我们的模型接受一个输入变量 `x`,并输出对应的函数值 `y`。我们使用 PyTorch 中的 `grad` 函数计算函数值 `y` 的一阶导数,并将它与 PDE 右边的函数 `f` 进行比较,得到模型的损失函数。我们还使用模型的输出和初值之间的误差作为另一项损失函数项。最后,我们使用 Adam 优化器来更新模型参数,训练模型,并在测试时绘制预测结果。
相关推荐
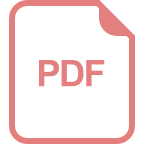














